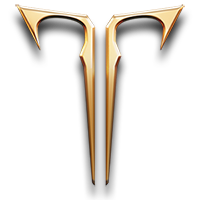 |
Lost Ark SDK
1.148.153.0
|
Go to the documentation of this file.
6 #pragma pack(push, 0x4)
17 #define CONST_RadToDeg 57.295779513082321600
18 #define CONST_InvAspectRatio16x9 0.56249
19 #define CONST_AspectRatio5x4 1.25
20 #define CONST_INDEX_NONE -1
21 #define CONST_InvAspectRatio5x4 0.8
22 #define CONST_AspectRatio16x9 1.77778
23 #define CONST_InvAspectRatio4x3 0.75
24 #define CONST_AspectRatio4x3 1.33333
25 #define CONST_UnrRotToDeg 0.00549316540360483
26 #define CONST_DegToUnrRot 182.0444
27 #define CONST_RadToUnrRot 10430.3783504704527
28 #define CONST_UnrRotToRad 0.00009587379924285
29 #define CONST_DegToRad 0.017453292519943296
30 #define CONST_Pi 3.1415926535897932
31 #define CONST_MaxInt 0x7fffffff
271 :
R(0),
G(0),
B(0),
A(0)
274 inline FColor(uint8_t r, uint8_t g, uint8_t b, uint8_t a)
EAutomatedRunResult
Definition: LA_Core_structs.hpp:48
float Y
Definition: LA_Core_structs.hpp:345
Definition: LA_Core_structs.hpp:236
int B
Definition: LA_Core_structs.hpp:713
Definition: LA_Core_structs.hpp:696
struct FVector4 ScaleShear
Definition: LA_Core_structs.hpp:468
@ LocObjectLanguageExtension_CHN
int D
Definition: LA_Core_structs.hpp:222
unsigned char Y
Definition: LA_Core_structs.hpp:606
unsigned char W
Definition: LA_Core_structs.hpp:608
@ IMT_UseFixedTangentEvalAndNewAutoTangents
Definition: LA_Core_structs.hpp:263
TArray< struct FInterpCurvePointVector > Points
Definition: LA_Core_structs.hpp:317
float A
Definition: LA_Core_structs.hpp:258
struct FSHVector G
Definition: LA_Core_structs.hpp:589
float G
Definition: LA_Core_structs.hpp:256
int SavedBulkDataOffsetInFile
Definition: LA_Core_structs.hpp:649
unsigned char UnknownData00[0xC]
Definition: LA_Core_structs.hpp:524
EFColMoveColOption
Definition: LA_Core_structs.hpp:133
unsigned char UnknownData00[0x1C]
Definition: LA_Core_structs.hpp:387
int ArrayNum
Definition: LA_Core_structs.hpp:616
int InlineHash
Definition: LA_Core_structs.hpp:682
Definition: LA_Core_structs.hpp:595
Definition: LA_Core_structs.hpp:304
class UDistributionVector * Distribution
Definition: LA_Core_structs.hpp:735
int NumPendingFences
Definition: LA_Core_structs.hpp:484
int A
Definition: LA_Core_structs.hpp:712
@ LocObjectLanguageExtension_RUS
struct FPlane YPlane
Definition: LA_Core_structs.hpp:362
struct FPointer VertexData
Definition: LA_Core_structs.hpp:625
EAxis
Definition: LA_Core_structs.hpp:91
float X
Definition: LA_Core_structs.hpp:247
EDebugBreakType
Definition: LA_Core_structs.hpp:38
TEnumAsByte< EInterpCurveMode > InterpMode
Definition: LA_Core_structs.hpp:508
@ IMT_UseFixedTangentEval
Definition: LA_Core_structs.hpp:533
Definition: LA_Core_structs.hpp:227
Definition: LA_Core_structs.hpp:436
struct FLinearColor OutVal
Definition: LA_Core_structs.hpp:505
Definition: LA_Core_structs.hpp:253
TEnumAsByte< EInterpMethodType > InterpMethod
Definition: LA_Core_structs.hpp:337
float InVal
Definition: LA_Core_structs.hpp:325
Definition: LA_Core_structs.hpp:725
float LookupTableStartTime
Definition: LA_Core_structs.hpp:497
int Dummy
Definition: LA_Core_structs.hpp:371
int SavedElementCount
Definition: LA_Core_structs.hpp:648
int C
Definition: LA_Core_structs.hpp:221
int BulkDataSizeOnDisk
Definition: LA_Core_structs.hpp:646
float Y
Definition: LA_Core_structs.hpp:248
TEnumAsByte< EInterpCurveMode > InterpMode
Definition: LA_Core_structs.hpp:528
TEnumAsByte< EInterpCurveMode > InterpMode
Definition: LA_Core_structs.hpp:310
@ AspectRatio_MajorAxisFOV
struct FQuat Rotation
Definition: LA_Core_structs.hpp:465
int B
Definition: LA_Core_structs.hpp:220
Definition: LA_Core_structs.hpp:444
struct FPointer Hash
Definition: LA_Core_structs.hpp:683
float Padding[0x3]
Definition: LA_Core_structs.hpp:581
EFColNavMeshOption
Definition: LA_Core_structs.hpp:115
Definition: LA_Core_structs.hpp:334
EInputEvent
Definition: LA_Core_structs.hpp:152
Definition: LA_Core_structs.hpp:456
Definition: LA_Core_structs.hpp:463
struct FLinearColor ArriveTangent
Definition: LA_Core_structs.hpp:506
Definition: LA_Core_structs.hpp:603
float R
Definition: LA_Core_structs.hpp:255
float AlphaTarget
Definition: LA_Core_structs.hpp:448
struct FVector Max
Definition: LA_Core_structs.hpp:563
struct FPointer Node
Definition: LA_Core_structs.hpp:476
float W
Definition: LA_Core_structs.hpp:354
Definition: LA_Core_structs.hpp:474
float OutVal
Definition: LA_Core_structs.hpp:326
class UDistributionFloat * Distribution
Definition: LA_Core_structs.hpp:720
float Z
Definition: LA_Core_structs.hpp:240
Definition: LA_Core_structs.hpp:489
struct FVector ArriveTangent
Definition: LA_Core_structs.hpp:308
int NumVertices
Definition: LA_Core_structs.hpp:628
unsigned char G
Definition: LA_Core_structs.hpp:266
struct FVector Location
Definition: LA_Core_structs.hpp:571
float SphereRadius
Definition: LA_Core_structs.hpp:431
EAlphaBlendType
Definition: LA_Core_structs.hpp:164
struct FLinearColor LeaveTangent
Definition: LA_Core_structs.hpp:507
struct FSHVector R
Definition: LA_Core_structs.hpp:588
float X
Definition: LA_Core_structs.hpp:238
int MaxBits
Definition: LA_Core_structs.hpp:664
Definition: LA_Core_structs.hpp:296
int Stride
Definition: LA_Core_structs.hpp:627
int X
Definition: LA_Core_structs.hpp:597
float MatineeValue
Definition: LA_Core_structs.hpp:727
Definition: LA_Core_structs.hpp:733
int A
Definition: LA_Core_structs.hpp:379
Definition: LA_Core_structs.hpp:359
@ LocObjectLanguageExtension_MAX
struct FVector4 Scale3D
Definition: LA_Core_structs.hpp:467
struct FPointer IndirectData
Definition: LA_Core_structs.hpp:661
Definition: LA_Core_structs.hpp:578
int bShouldFreeOnEmpty
Definition: LA_Core_structs.hpp:654
int SavedBulkDataSizeOnDisk
Definition: LA_Core_structs.hpp:650
Definition: LA_Core_structs.hpp:552
@ LocObjectLanguageExtension_INT
int FirstFreeIndex
Definition: LA_Core_structs.hpp:673
@ AspectRatio_MaintainYFOV
TEnumAsByte< EInterpMethodType > InterpMethod
Definition: LA_Core_structs.hpp:536
Definition: LA_Core_structs.hpp:633
FColor(uint8_t r, uint8_t g, uint8_t b, uint8_t a)
Definition: LA_Core_structs.hpp:274
int BulkDataOffsetInFile
Definition: LA_Core_structs.hpp:645
int ElementCount
Definition: LA_Core_structs.hpp:644
TEnumAsByte< EInterpMethodType > InterpMethod
Definition: LA_Core_structs.hpp:299
int NumFreeIndices
Definition: LA_Core_structs.hpp:674
int Value
Definition: LA_Core_structs.hpp:705
int Data
Definition: LA_Core_structs.hpp:626
TArray< float > LookupTable
Definition: LA_Core_structs.hpp:495
float B
Definition: LA_Core_structs.hpp:257
EFColMovePickingOption
Definition: LA_Core_structs.hpp:142
int InlineData[0x4]
Definition: LA_Core_structs.hpp:662
struct FVector v1
Definition: LA_Core_structs.hpp:438
struct FSet_Mirror Pairs
Definition: LA_Core_structs.hpp:698
Definition: LA_Core_structs.hpp:669
TEnumAsByte< EInterpCurveMode > InterpMode
Definition: LA_Core_structs.hpp:329
TArray< struct FInterpCurvePointVector2D > Points
Definition: LA_Core_structs.hpp:298
int NumBits
Definition: LA_Core_structs.hpp:663
float Y
Definition: LA_Core_structs.hpp:239
float ArriveTangent
Definition: LA_Core_structs.hpp:327
EFColProjectileOption
Definition: LA_Core_structs.hpp:124
unsigned char UnknownData00[0x4]
Definition: LA_Core_structs.hpp:372
float LeaveTangent
Definition: LA_Core_structs.hpp:328
float Y
Definition: LA_Core_structs.hpp:412
struct FTwoVectors OutVal
Definition: LA_Core_structs.hpp:544
float FOV
Definition: LA_Core_structs.hpp:573
float LookupTableTimeScale
Definition: LA_Core_structs.hpp:496
int Y
Definition: LA_Core_structs.hpp:598
Definition: LA_Core_structs.hpp:285
Definition: LA_Core_structs.hpp:392
int B
Definition: LA_Core_structs.hpp:380
float Z
Definition: LA_Core_structs.hpp:413
unsigned char LookupTableChunkSize
Definition: LA_Core_structs.hpp:494
Definition: LA_Core_structs.hpp:352
struct FPointer BulkData
Definition: LA_Core_structs.hpp:651
ETickingGroup
Definition: LA_Core_structs.hpp:103
struct FPointer VfTable
Definition: LA_Core_structs.hpp:624
unsigned char Type
Definition: LA_Core_structs.hpp:491
Definition: LA_Core_structs.hpp:679
Definition: LA_Core_structs.hpp:385
int Roll
Definition: LA_Core_structs.hpp:231
struct FSparseArray_Mirror Elements
Definition: LA_Core_structs.hpp:681
int ElementIndex
Definition: LA_Core_structs.hpp:477
int ArrayMax
Definition: LA_Core_structs.hpp:396
Definition: LA_Core_structs.hpp:541
struct FSet_Mirror Pairs
Definition: LA_Core_structs.hpp:691
struct FVector2D ArriveTangent
Definition: LA_Core_structs.hpp:289
unsigned char A
Definition: LA_Core_structs.hpp:268
float AlphaIn
Definition: LA_Core_structs.hpp:446
Definition: LA_Core_structs.hpp:640
struct FTwoVectors ArriveTangent
Definition: LA_Core_structs.hpp:545
float V[0x9]
Definition: LA_Core_structs.hpp:580
Definition: LA_Core_structs.hpp:369
Definition: LA_Core_structs.hpp:342
int NumPendingFences
Definition: LA_Core_structs.hpp:635
Definition: LA_Core_structs.hpp:521
Definition: LA_AkAudio_classes.hpp:11
unsigned char X
Definition: LA_Core_structs.hpp:605
Definition: LA_Core_structs.hpp:659
Definition: LA_Core_structs.hpp:409
struct FVector BoxExtent
Definition: LA_Core_structs.hpp:430
int HashSize
Definition: LA_Core_structs.hpp:684
struct FPlane WPlane
Definition: LA_Core_structs.hpp:364
int BulkDataFlags
Definition: LA_Core_structs.hpp:643
float Radius
Definition: LA_Core_structs.hpp:421
unsigned char LookupTableNumElements
Definition: LA_Core_structs.hpp:493
unsigned char R
Definition: LA_Core_structs.hpp:267
struct FPlane ZPlane
Definition: LA_Core_structs.hpp:363
float InVal
Definition: LA_Core_structs.hpp:543
TEnumAsByte< EInterpCurveMode > InterpMode
Definition: LA_Core_structs.hpp:291
Definition: LA_Core_structs.hpp:613
struct FPointer Data
Definition: LA_Core_structs.hpp:394
struct FVector v2
Definition: LA_Core_structs.hpp:439
Definition: LA_Core_structs.hpp:569
Definition: LA_Core_structs.hpp:718
float W
Definition: LA_Core_structs.hpp:414
TArray< struct FInterpCurvePointQuat > Points
Definition: LA_Core_structs.hpp:535
int Pitch
Definition: LA_Core_structs.hpp:229
struct FRotator Rotation
Definition: LA_Core_structs.hpp:572
struct FVector2D LeaveTangent
Definition: LA_Core_structs.hpp:290
TEnumAsByte< EInterpCurveMode > InterpMode
Definition: LA_Core_structs.hpp:547
TArray< struct FInterpCurvePointFloat > Points
Definition: LA_Core_structs.hpp:336
Definition: LA_Core_classes.hpp:1111
struct FQuat LeaveTangent
Definition: LA_Core_structs.hpp:527
TArray< struct FInterpCurvePointLinearColor > Points
Definition: LA_Core_structs.hpp:515
int ArrayMax
Definition: LA_Core_structs.hpp:617
Definition: LA_Core_structs.hpp:377
struct FPointer Data
Definition: LA_Core_structs.hpp:615
struct FPointer InlineData
Definition: LA_Core_structs.hpp:403
Definition: LA_Core_structs.hpp:323
float InVal
Definition: LA_Core_structs.hpp:306
Definition: LA_Core_structs.hpp:427
Definition: LA_Core_structs.hpp:217
EInterpCurveMode
Definition: LA_Core_structs.hpp:68
float W
Definition: LA_Core_structs.hpp:347
unsigned long bInMatinee
Definition: LA_Core_structs.hpp:728
struct FVector Origin
Definition: LA_Core_structs.hpp:429
struct FQuat OutVal
Definition: LA_Core_structs.hpp:525
unsigned char Op
Definition: LA_Core_structs.hpp:492
struct FQuat ArriveTangent
Definition: LA_Core_structs.hpp:526
float Height
Definition: LA_Core_structs.hpp:422
TArray< int > Elements
Definition: LA_Core_structs.hpp:671
struct FVector2D OutVal
Definition: LA_Core_structs.hpp:288
struct FVector OutVal
Definition: LA_Core_structs.hpp:307
EDistributionVectorMirrorFlags
Definition: LA_Core_structs.hpp:190
TEnumAsByte< EInterpMethodType > InterpMethod
Definition: LA_Core_structs.hpp:516
float InVal
Definition: LA_Core_structs.hpp:287
float Z
Definition: LA_Core_structs.hpp:346
int A
Definition: LA_Core_structs.hpp:458
struct FVector LeaveTangent
Definition: LA_Core_structs.hpp:309
unsigned char B
Definition: LA_Core_structs.hpp:265
float X
Definition: LA_Core_structs.hpp:411
int A
Definition: LA_Core_structs.hpp:219
FColor()
Definition: LA_Core_structs.hpp:270
Definition: LA_Core_structs.hpp:245
float AlphaOut
Definition: LA_Core_structs.hpp:447
struct FSHVector B
Definition: LA_Core_structs.hpp:590
struct FBitArray_Mirror AllocationFlags
Definition: LA_Core_structs.hpp:672
Definition: LA_Core_structs.hpp:502
@ AspectRatio_MaintainXFOV
unsigned char IsValid
Definition: LA_Core_structs.hpp:564
struct FPointer AttachedAr
Definition: LA_Core_structs.hpp:653
TEnumAsByte< EInterpMethodType > InterpMethod
Definition: LA_Core_structs.hpp:318
float X
Definition: LA_Core_structs.hpp:344
Definition: LA_Basic.hpp:22
Definition: LA_Core_structs.hpp:401
TArray< struct FInterpCurvePointTwoVectors > Points
Definition: LA_Core_structs.hpp:554
int bScaleInheritance
Definition: LA_Core_structs.hpp:469
struct FVector4 Translation
Definition: LA_Core_structs.hpp:466
Definition: LA_Core_structs.hpp:622
struct FArray_Mirror SecondaryData
Definition: LA_Core_structs.hpp:404
Definition: LA_Core_structs.hpp:315
int SavedBulkDataFlags
Definition: LA_Core_structs.hpp:647
TEnumAsByte< EAlphaBlendType > BlendType
Definition: LA_Core_structs.hpp:451
@ LocObjectLanguageExtension_JPN
Definition: LA_Core_structs.hpp:586
Definition: LA_Core_structs.hpp:710
float InVal
Definition: LA_Core_structs.hpp:523
float BlendTime
Definition: LA_Core_structs.hpp:449
int Yaw
Definition: LA_Core_structs.hpp:230
float BlendTimeToGo
Definition: LA_Core_structs.hpp:450
EDistributionVectorLockFlags
Definition: LA_Core_structs.hpp:178
struct FVector Min
Definition: LA_Core_structs.hpp:562
EInterpMethodType
Definition: LA_Core_structs.hpp:81
ELocObjectLanguageExtension
Definition: LA_Core_structs.hpp:200
struct FPlane XPlane
Definition: LA_Core_structs.hpp:361
Definition: LA_Core_classes.hpp:1133
Definition: LA_Core_structs.hpp:419
float InVal
Definition: LA_Core_structs.hpp:504
Definition: LA_Core_structs.hpp:703
Definition: LA_Core_structs.hpp:482
Definition: LA_Core_structs.hpp:689
@ IMT_UseBrokenTangentEval
struct FTwoVectors LeaveTangent
Definition: LA_Core_structs.hpp:546
int ArrayNum
Definition: LA_Core_structs.hpp:395
Definition: LA_Core_structs.hpp:513
int LockStatus
Definition: LA_Core_structs.hpp:652
TEnumAsByte< EInterpMethodType > InterpMethod
Definition: LA_Core_structs.hpp:555
EAspectRatioAxisConstraint
Definition: LA_Core_structs.hpp:58
unsigned char Z
Definition: LA_Core_structs.hpp:607
struct FPointer VfTable
Definition: LA_Core_structs.hpp:642
Definition: LA_Core_structs.hpp:560