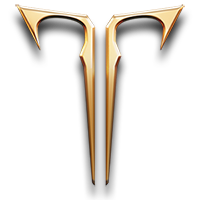 |
Lost Ark SDK
1.148.153.0
|
Go to the documentation of this file.
6 #pragma pack(push, 0x4)
9 #include <unordered_set>
17 auto vtable = *
reinterpret_cast<const void***
>(
const_cast<void*
>(instance));
18 return reinterpret_cast<Fn
>(vtable[index]);
33 inline size_t Num()
const
78 Max =
Count = *other ? std::wcslen(other) + 1 : 0;
82 Data =
const_cast<wchar_t*
>(other);
88 return Data !=
nullptr;
91 inline const wchar_t*
c_str()
const
98 const auto length = std::wcslen(
Data);
100 std::string str(length,
'\0');
102 std::use_facet<std::ctype<wchar_t>>(std::locale()).narrow(
Data,
Data + length,
'?', &str[0]);
108 template<
class TEnum>
117 :
value(static_cast<uint8_t>(_value))
122 :
value(static_cast<uint8_t>(_value))
131 inline operator TEnum()
const
178 static std::unordered_set<size_t> cache;
248 template<
class InterfaceType>
262 inline operator bool()
const
int32_t Max
Definition: LA_Basic.hpp:66
int32_t Number
Definition: LA_Basic.hpp:160
char name[0x14]
Definition: LA_Basic.hpp:149
Definition: LA_Basic.hpp:225
bool IsValidIndex(size_t i) const
Definition: LA_Basic.hpp:48
TEnumAsByte(int32_t _value)
Definition: LA_Basic.hpp:121
UObject *& GetObjectRef()
Definition: LA_Basic.hpp:237
void * InterfacePointer
Definition: LA_Basic.hpp:229
Definition: LA_Basic.hpp:69
T & GetByIndex(size_t i)
Definition: LA_Basic.hpp:53
static TArray< FNameEntry * > * GNames
Definition: LA_Basic.hpp:204
Fn GetVFunction(const void *instance, std::size_t index)
Definition: LA_Basic.hpp:15
bool IsValid() const
Definition: LA_Basic.hpp:86
std::string ToString() const
Definition: LA_Basic.hpp:96
TArray()
Definition: LA_Basic.hpp:27
InterfaceType & operator*() const
Definition: LA_Basic.hpp:257
const T & operator[](size_t i) const
Definition: LA_Basic.hpp:43
int32_t Count
Definition: LA_Basic.hpp:65
const T & GetByIndex(size_t i) const
Definition: LA_Basic.hpp:58
FName(int32_t i)
Definition: LA_Basic.hpp:168
Definition: LA_Basic.hpp:249
bool operator==(const FName &other) const
Definition: LA_Basic.hpp:217
FString(const wchar_t *other)
Definition: LA_Basic.hpp:76
struct FName FunctionName
Definition: LA_Basic.hpp:271
FName()
Definition: LA_Basic.hpp:162
int32_t Index
Definition: LA_Basic.hpp:159
Definition: LA_Basic.hpp:157
Definition: LA_Basic.hpp:268
static TArray< FNameEntry * > & GetGlobalNames()
Definition: LA_Basic.hpp:207
FName(const char *nameToFind)
Definition: LA_Basic.hpp:174
std::string GetName() const
Definition: LA_Basic.hpp:151
char UnknownData00[0x14]
Definition: LA_Basic.hpp:148
InterfaceType * operator->() const
Definition: LA_Basic.hpp:252
Definition: LA_AkAudio_classes.hpp:11
TEnum GetValue() const
Definition: LA_Basic.hpp:136
class UObject * Object
Definition: LA_Basic.hpp:270
Definition: LA_Core_classes.hpp:19
UObject * ObjectPointer
Definition: LA_Basic.hpp:228
FString()
Definition: LA_Basic.hpp:72
UObject * GetObject() const
Definition: LA_Basic.hpp:232
void * GetInterface() const
Definition: LA_Basic.hpp:242
Definition: LA_Basic.hpp:145
T * Data
Definition: LA_Basic.hpp:64
const wchar_t * c_str() const
Definition: LA_Basic.hpp:91
Definition: LA_Basic.hpp:22
TEnumAsByte(TEnum _value)
Definition: LA_Basic.hpp:116
TEnumAsByte()
Definition: LA_Basic.hpp:112
std::string GetName() const
Definition: LA_Basic.hpp:212
T & operator[](size_t i)
Definition: LA_Basic.hpp:38
uint8_t value
Definition: LA_Basic.hpp:142
size_t Num() const
Definition: LA_Basic.hpp:33
TEnumAsByte(uint8_t _value)
Definition: LA_Basic.hpp:126
Definition: LA_Basic.hpp:109