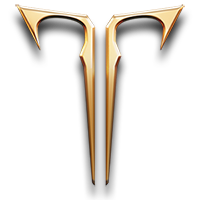 |
Lost Ark SDK
1.148.153.0
|
Go to the documentation of this file.
6 #pragma pack(push, 0x4)
59 if (
object ==
nullptr)
64 if (object->GetFullName() == name)
66 return static_cast<T*
>(object);
74 return FindObject<UClass>(name);
87 static UClass* ptr =
nullptr;
95 return GetVFunction<void(__thiscall *)(UObject*, class UFunction*, void*)>(
this, 67)(
this,
function, parms);
113 void GetSystemTime(
int* Year,
int* Month,
int* DayOfWeek,
int* Day,
int* Hour,
int* Min,
int* Sec,
int* MSec);
118 float ByteToFloat(
unsigned char inputByte,
bool bSigned);
119 unsigned char FloatToByte(
float inputFloat,
bool bSigned);
152 bool IsInState(
const struct FName& TestState,
bool bTestStateStack);
153 void GotoState(
const struct FName& NewState,
const struct FName& Label,
bool bForceEvents,
bool bKeepStack);
212 bool IsA(
const struct FName& ClassName);
247 bool STATIC_SClampRotAxis(
float DeltaTime,
int ViewAxis,
int MaxLimit,
int MinLimit,
float InterpolationSpeed,
int* out_DeltaViewAxis);
250 void STATIC_ClampRotAxis(
int ViewAxis,
int MaxLimit,
int MinLimit,
int* out_DeltaViewAxis);
311 float STATIC_FInterpTo(
float Current,
float Target,
float DeltaTime,
float InterpSpeed);
415 static UClass* ptr =
nullptr;
433 static UClass* ptr =
nullptr;
469 static UClass* ptr =
nullptr;
487 static UClass* ptr =
nullptr;
505 static UClass* ptr =
nullptr;
523 static UClass* ptr =
nullptr;
541 static UClass* ptr =
nullptr;
559 static UClass* ptr =
nullptr;
577 static UClass* ptr =
nullptr;
595 static UClass* ptr =
nullptr;
613 static UClass* ptr =
nullptr;
630 static UClass* ptr =
nullptr;
648 static UClass* ptr =
nullptr;
670 static UClass* ptr =
nullptr;
688 static UClass* ptr =
nullptr;
714 static UClass* ptr =
nullptr;
732 static UClass* ptr =
nullptr;
750 static UClass* ptr =
nullptr;
767 static UClass* ptr =
nullptr;
785 static UClass* ptr =
nullptr;
802 static UClass* ptr =
nullptr;
820 static UClass* ptr =
nullptr;
837 static UClass* ptr =
nullptr;
855 static UClass* ptr =
nullptr;
873 static UClass* ptr =
nullptr;
891 static UClass* ptr =
nullptr;
908 static UClass* ptr =
nullptr;
926 static UClass* ptr =
nullptr;
944 static UClass* ptr =
nullptr;
962 static UClass* ptr =
nullptr;
980 static UClass* ptr =
nullptr;
998 static UClass* ptr =
nullptr;
1016 static UClass* ptr =
nullptr;
1043 static UClass* ptr =
nullptr;
1060 static UClass* ptr =
nullptr;
1081 static UClass* ptr =
nullptr;
1100 static UClass* ptr =
nullptr;
1120 static UClass* ptr =
nullptr;
1142 static UClass* ptr =
nullptr;
1171 static UClass* ptr =
nullptr;
1190 static UClass* ptr =
nullptr;
1210 static UClass* ptr =
nullptr;
1228 static UClass* ptr =
nullptr;
1246 template<
typename T>
1255 static Fn fn =
nullptr;
1265 static UClass* ptr =
nullptr;
unsigned char UnknownData01[0x44]
Definition: LA_Core_classes.hpp:666
struct FQuat STATIC_QuatSlerp(const struct FQuat &A, const struct FQuat &B, float Alpha, bool bShortestPath)
bool STATIC_NotEqual_NameName(const struct FName &A, const struct FName &B)
TArray< struct FString > LocalizationPaths
Definition: LA_Core_classes.hpp:464
class UObject * ObjectArchetype
Definition: LA_Core_classes.hpp:33
FName FriendlyName
Definition: LA_Core_classes.hpp:704
struct FString STATIC_Concat_StrStr(const struct FString &A, const struct FString &B)
Definition: LA_Core_classes.hpp:1239
struct FVector STATIC_VRandCone2(const struct FVector &Dir, float HorizontalConeHalfAngleRadians, float VerticalConeHalfAngleRadians)
bool STATIC_EqualEqual_ObjectObject(class UObject *A, class UObject *B)
unsigned char UnknownData00[0x8]
Definition: LA_Core_classes.hpp:519
struct FVector2D STATIC_AddEqual_Vector2DVector2D(const struct FVector2D &B, struct FVector2D *A)
float STATIC_Loge(float A)
Definition: LA_Core_structs.hpp:236
struct FRotator STATIC_MultiplyEqual_RotatorFloat(float B, struct FRotator *A)
unsigned char UnknownData00[0x8]
Definition: LA_Core_classes.hpp:976
float STATIC_Subtract_PreFloat(float A)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:818
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:1226
struct FString STATIC_AtEqual_StrStr(const struct FString &B, struct FString *A)
int32_t Number
Definition: LA_Basic.hpp:160
struct FColor STATIC_Multiply_ColorFloat(const struct FColor &A, float B)
struct FString STATIC_Chr(int I)
int STATIC_AddAdd_PreInt(int *A)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:431
Definition: LA_Core_classes.hpp:498
Definition: LA_Core_classes.hpp:408
int STATIC_ClampRotAxisFromRange(int Current, int Min, int Max)
uint8_t NumParms
Definition: LA_Core_classes.hpp:707
Definition: LA_Core_structs.hpp:263
struct FRotator STATIC_Add_RotatorRotator(const struct FRotator &A, const struct FRotator &B)
float STATIC_GetMappedRangeValue(const struct FVector2D &InputRange, const struct FVector2D &OutputRange, float Value)
float STATIC_NoZDot(const struct FVector &A, const struct FVector &B)
unsigned char UnknownData00[0x8]
Definition: LA_Core_classes.hpp:816
int STATIC_SubtractSubtract_Int(int *A)
struct FPointer VfTable_FExec
Definition: LA_Core_classes.hpp:429
Definition: LA_Core_classes.hpp:659
int STATIC_GreaterGreater_IntInt(int A, int B)
bool STATIC_SClampRotAxis(float DeltaTime, int ViewAxis, int MaxLimit, int MinLimit, float InterpolationSpeed, int *out_DeltaViewAxis)
Definition: LA_Core_classes.hpp:606
bool STATIC_ComplementEqual_FloatFloat(float A, float B)
bool STATIC_GreaterEqual_IntInt(int A, int B)
struct FRotator STATIC_MakeRotator(int Pitch, int Yaw, int Roll)
float STATIC_FindDeltaAngle(float A1, float A2)
bool STATIC_NotEqual_StrStr(const struct FString &A, const struct FString &B)
unsigned char UnknownData00[0x10]
Definition: LA_Core_classes.hpp:922
void STATIC_GetAxes(const struct FRotator &A, struct FVector *X, struct FVector *Y, struct FVector *Z)
void STATIC_GetAngularFromDotDist(const struct FVector2D &DotDist, struct FVector2D *OutAngDist)
struct FVector2D STATIC_Add_Vector2DVector2D(const struct FVector2D &A, const struct FVector2D &B)
float STATIC_Add_FloatFloat(float A, float B)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:942
Definition: LA_Core_classes.hpp:902
int STATIC_Subtract_PreInt(int A)
float STATIC_SubtractEqual_FloatFloat(float B, float *A)
float STATIC_Percent_FloatFloat(float A, float B)
bool IsA(UClass *cmp) const
unsigned char UnknownData00[0x8]
Definition: LA_Core_classes.hpp:1074
struct FVector STATIC_Multiply_FloatVector(float A, const struct FVector &B)
bool STATIC_Greater_IntInt(int A, int B)
Definition: LA_Core_classes.hpp:480
TArray< struct FString > HelpParamDescriptions
Definition: LA_Core_classes.hpp:1162
bool STATIC_NotEqual_FloatFloat(float A, float B)
float STATIC_Sin(float A)
struct FName STATIC_GetFuncName()
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:1058
bool STATIC_AndAnd_BoolBool(bool A, bool B)
bool STATIC_EqualEqual_RotatorRotator(const struct FRotator &A, const struct FRotator &B)
struct FString STATIC_Right(const struct FString &S, int I)
float STATIC_GetHeadingAngle(const struct FVector &Dir)
Definition: LA_Core_classes.hpp:588
Definition: LA_Core_structs.hpp:227
struct FVector STATIC_SubtractEqual_VectorVector(const struct FVector &B, struct FVector *A)
struct FName Name
Definition: LA_Core_classes.hpp:31
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:1041
Definition: LA_Core_structs.hpp:253
float STATIC_Acos(float A)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:539
float STATIC_Tan(float A)
struct FString SavePath
Definition: LA_Core_classes.hpp:452
uint16_t PropertySize
Definition: LA_Core_classes.hpp:665
Definition: LA_Core_classes.hpp:1071
int AutoPriority
Definition: LA_Core_classes.hpp:1038
void STATIC_StaticSaveConfig()
struct FRotator STATIC_Subtract_RotatorRotator(const struct FRotator &A, const struct FRotator &B)
int STATIC_NormalizeRotAxis(int Angle)
static T * FindObject(const std::string &name)
Definition: LA_Core_classes.hpp:53
Definition: LA_Core_classes.hpp:937
struct FVector STATIC_ClampLength(const struct FVector &V, float MaxLength)
unsigned long bText
Definition: LA_Core_classes.hpp:1037
struct FVector STATIC_VRand()
int Main(const struct FString &Params)
struct FVector2D STATIC_Subtract_Vector2DVector2D(const struct FVector2D &A, const struct FVector2D &B)
static TArray< UObject * > & GetGlobalObjects()
Definition: LA_Core_classes.hpp:35
int STATIC_Add_IntInt(int A, int B)
int STATIC_InStr(const struct FString &S, const struct FString &T, bool bSearchFromRight, bool bIgnoreCase, int StartPos)
struct FLinearColor STATIC_Subtract_LinearColorLinearColor(const struct FLinearColor &A, const struct FLinearColor &B)
float STATIC_RandRange(float InMin, float InMax)
int StaleCacheDays
Definition: LA_Core_classes.hpp:447
void STATIC_ProfNodeStop(int AssumedTimerIndex)
void STATIC_ParseStringIntoArray(const struct FString &BaseString, const struct FString &delim, bool bCullEmpty, TArray< struct FString > *Pieces)
struct FString TextureFileCacheExtension
Definition: LA_Core_classes.hpp:465
struct FVector STATIC_EvalInterpCurveVector(float InVal, struct FInterpCurveVector *VectorCurve)
unsigned long bEditorImport
Definition: LA_Core_classes.hpp:1036
struct FVector STATIC_MatrixGetAxis(const struct FMatrix &TM, TEnumAsByte< EAxis > Axis)
bool STATIC_NotEqual_ObjectObject(class UObject *A, class UObject *B)
struct FString STATIC_Left(const struct FString &S, int I)
unsigned char UnknownData00[0x4]
Definition: LA_Core_classes.hpp:703
UObject * CreateDefaultObject()
Definition: LA_Core_classes.hpp:1252
int STATIC_ClampRotAxisFromBase(int Current, int Center, int MaxDelta)
Definition: LA_Core_structs.hpp:334
TArray< struct FString > SeekFreePCPaths
Definition: LA_Core_classes.hpp:457
struct FRotator STATIC_AddEqual_RotatorRotator(const struct FRotator &B, struct FRotator *A)
unsigned long bIsDirty
Definition: LA_Core_classes.hpp:1138
Definition: LA_Basic.hpp:69
struct FVector2D STATIC_Divide_Vector2DFloat(const struct FVector2D &A, float B)
TArray< struct FString > HelpParamNames
Definition: LA_Core_classes.hpp:1161
struct FVector STATIC_Multiply_VectorVector(const struct FVector &A, const struct FVector &B)
unsigned char STATIC_MultiplyEqual_ByteFloat(float B, unsigned char *A)
bool InCylinder(const struct FVector &Origin, const struct FRotator &Dir, float Width, const struct FVector &A, bool bIgnoreZ)
struct FRotator STATIC_SubtractEqual_RotatorRotator(const struct FRotator &B, struct FRotator *A)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:730
struct FLinearColor STATIC_ColorToLinearColor(const struct FColor &OldColor)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:978
struct FQuat STATIC_Subtract_QuatQuat(const struct FQuat &A, const struct FQuat &B)
int STATIC_GreaterGreaterGreater_IntInt(int A, int B)
unsigned long bIsDirty
Definition: LA_Core_classes.hpp:1116
TArray< struct FString > SeekFreePCExtensions
Definition: LA_Core_classes.hpp:463
struct FString CachePath
Definition: LA_Core_classes.hpp:453
Definition: LA_Core_classes.hpp:1027
uint16_t iNative
Definition: LA_Core_classes.hpp:705
int STATIC_Multiply_IntInt(int A, int B)
bool STATIC_IsGuidValid(struct FGuid *InGuid)
struct FRotator STATIC_Divide_RotatorFloat(const struct FRotator &A, float B)
Definition: LA_Core_classes.hpp:955
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:712
std::string UObject::GetName() const
Definition: LA_Core_classes.hpp:40
int STATIC_AddAdd_Int(int *A)
struct FLinearColor STATIC_MakeLinearColor(float R, float G, float B, float A)
int STATIC_Divide_IntInt(int A, int B)
int STATIC_FCeil(float A)
Definition: LA_Core_classes.hpp:761
unsigned char STATIC_MultiplyEqual_ByteByte(unsigned char B, unsigned char *A)
bool STATIC_GreaterEqual_FloatFloat(float A, float B)
struct FLinearColor STATIC_Multiply_LinearColorFloat(const struct FLinearColor &LC, float Mult)
int STATIC_AddEqual_IntInt(int B, int *A)
unsigned long bEditAfterNew
Definition: LA_Core_classes.hpp:1035
unsigned char UnknownData00[0x10]
Definition: LA_Core_classes.hpp:851
void STATIC_ProfNodeEvent(const struct FString &EventName)
bool STATIC_NotEqual_BoolBool(bool A, bool B)
int ObjectInternalInteger
Definition: LA_Core_classes.hpp:28
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:783
int STATIC_Asc(const struct FString &S)
float STATIC_FClamp(float V, float A, float B)
bool STATIC_EqualEqual_StrStr(const struct FString &A, const struct FString &B)
float STATIC_Multiply_FloatFloat(float A, float B)
struct FVector STATIC_AddEqual_VectorVector(const struct FVector &B, struct FVector *A)
uint16_t * ReturnValueOffset
Definition: LA_Core_classes.hpp:709
int STATIC_Len(const struct FString &S)
struct FRotator STATIC_Multiply_RotatorFloat(const struct FRotator &A, float B)
bool STATIC_XorXor_BoolBool(bool A, bool B)
int GetRandomOptionSumFrequency(TArray< float > *FreqList)
unsigned char UnknownData00[0x168]
Definition: LA_Core_classes.hpp:573
static T * GetObjectCasted(std::size_t index)
Definition: LA_Core_classes.hpp:78
TArray< struct FString > ScriptPaths
Definition: LA_Core_classes.hpp:458
struct FQuat STATIC_QuatFindBetween(const struct FVector &A, const struct FVector &B)
struct FString STATIC_SubtractEqual_StrStr(const struct FString &B, struct FString *A)
struct FQuat STATIC_QuatProduct(const struct FQuat &A, const struct FQuat &B)
TArray< struct FString > Formats
Definition: LA_Core_classes.hpp:1033
float STATIC_EvalInterpCurveFloat(float InVal, struct FInterpCurveFloat *FloatCurve)
struct FRotator STATIC_MatrixGetRotator(const struct FMatrix &TM)
struct FVector STATIC_Multiply_VectorFloat(const struct FVector &A, float B)
struct FVector STATIC_MultiplyEqual_VectorVector(const struct FVector &B, struct FVector *A)
float STATIC_MultiplyMultiply_FloatFloat(float Base, float Exp)
unsigned long IsServer
Definition: LA_Core_classes.hpp:1163
struct FVector2D STATIC_SubtractEqual_Vector2DVector2D(const struct FVector2D &B, struct FVector2D *A)
struct FString STATIC_GetStringFromGuid(struct FGuid *InGuid)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:1098
float STATIC_Atan(float A)
TArray< struct FName > Suppress
Definition: LA_Core_classes.hpp:461
float STATIC_FMin(float A, float B)
UObject * ClassDefaultObject
Definition: LA_Core_classes.hpp:1243
Definition: LA_Core_classes.hpp:1054
float STATIC_FInterpTo(float Current, float Target, float DeltaTime, float InterpSpeed)
unsigned char UnknownData00[0x8]
Definition: LA_Core_classes.hpp:940
Definition: LA_Core_structs.hpp:296
int STATIC_Percent_IntInt(int A, int B)
struct FColor STATIC_Multiply_FloatColor(float A, const struct FColor &B)
struct FVector STATIC_Cross_VectorVector(const struct FVector &A, const struct FVector &B)
bool STATIC_NotEqual_IntInt(int A, int B)
int STATIC_EnumFromString(class UObject *E, const struct FName &ValueName)
void ProcessEvent(class UFunction *function, void *parms)
Definition: LA_Core_classes.hpp:93
struct FPointer VfTable_FCurveEdInterface
Definition: LA_Core_classes.hpp:1114
unsigned char UnknownData00[0x10]
Definition: LA_Core_classes.hpp:662
void STATIC_WarnInternal(const struct FString &S)
Definition: LA_Core_structs.hpp:359
struct FColor STATIC_LerpColor(const struct FColor &A, const struct FColor &B, float Alpha)
struct FString STATIC_ConcatEqual_StrStr(const struct FString &B, struct FString *A)
int STATIC_Subtract_IntInt(int A, int B)
void Disable(const struct FName &ProbeFunc)
unsigned char UnknownData00[0x54]
Definition: LA_Core_classes.hpp:1224
struct FName GetPackageName()
unsigned long LogToConsole
Definition: LA_Core_classes.hpp:1166
struct FVector STATIC_ProjectOnTo(const struct FVector &X, const struct FVector &Y)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:467
void STATIC_SetUTracing(bool bShouldUTrace)
unsigned char STATIC_AddAdd_Byte(unsigned char *A)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:800
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:686
Definition: LA_Basic.hpp:249
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:575
bool STATIC_NotEqual_InterfaceInterface(const TScriptInterface< class UInterface > &A, const TScriptInterface< class UInterface > &B)
struct FQWord ObjectFlags
Definition: LA_Core_classes.hpp:24
TArray< struct FString > FRScriptPaths
Definition: LA_Core_classes.hpp:459
struct FString STATIC_Split(const struct FString &Text, const struct FString &SplitStr, bool bOmitSplitStr)
void STATIC_LogInternal(const struct FString &S, const struct FName &Tag)
bool STATIC_EqualEqual_VectorVector(const struct FVector &A, const struct FVector &B)
struct FVector STATIC_VRandCone(const struct FVector &Dir, float ConeHalfAngleRadians)
Definition: LA_Core_classes.hpp:681
Definition: LA_Core_classes.hpp:991
bool STATIC_Less_StrStr(const struct FString &A, const struct FString &B)
int NetIndex
Definition: LA_Core_classes.hpp:29
Definition: LA_Core_classes.hpp:831
int STATIC_ProfNodeStart(const struct FString &TimerName)
bool STATIC_NotEqual_VectorVector(const struct FVector &A, const struct FVector &B)
Definition: LA_Core_classes.hpp:866
unsigned long bCanBeBaked
Definition: LA_Core_classes.hpp:1115
Definition: LA_Core_classes.hpp:884
unsigned char STATIC_SubtractSubtract_PreByte(unsigned char *A)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:960
Definition: LA_Core_classes.hpp:1203
float STATIC_FCubicInterp(float P0, float T0, float P1, float T1, float A)
void BeginState(const struct FName &PreviousStateName)
int Main(const struct FString &Params)
unsigned char UnknownData00[0x10]
Definition: LA_Core_classes.hpp:1012
class UClass * Class
Definition: LA_Core_classes.hpp:32
int STATIC_Round(float A)
struct FPointer VfTableObject
Definition: LA_Core_classes.hpp:23
unsigned char UnknownData00[0x24]
Definition: LA_Core_classes.hpp:684
struct FRotator STATIC_RTransform(const struct FRotator &R, const struct FRotator &RBasis)
float STATIC_GetRangePctByValue(const struct FVector2D &Range, float Value)
struct FString CacheExt
Definition: LA_Core_classes.hpp:454
struct FColor STATIC_Subtract_ColorColor(const struct FColor &A, const struct FColor &B)
void STATIC_ClampRotAxis(int ViewAxis, int MaxLimit, int MinLimit, int *out_DeltaViewAxis)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:1169
struct FColor STATIC_MakeColor(unsigned char R, unsigned char G, unsigned char B, unsigned char A)
float STATIC_GetRangeValueByPct(const struct FVector2D &Range, float Pct)
struct FGuid STATIC_CreateGuid()
struct FVector STATIC_MirrorVectorByNormal(const struct FVector &InVect, const struct FVector &InNormal)
class UField * Next
Definition: LA_Core_classes.hpp:644
unsigned char UnknownData00[0x610]
Definition: LA_Core_classes.hpp:609
struct FQuat STATIC_QuatFromRotator(const struct FRotator &A)
void STATIC_ProfNodeSetDepthThreshold(int Depth)
struct FPointer StateFrame
Definition: LA_Core_classes.hpp:25
bool STATIC_Less_IntInt(int A, int B)
int STATIC_SubtractEqual_IntInt(int B, int *A)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:521
bool STATIC_GetDotDistance(const struct FVector &Direction, const struct FVector &AxisX, const struct FVector &AxisY, const struct FVector &AxisZ, struct FVector2D *OutDotDist)
unsigned long IsClient
Definition: LA_Core_classes.hpp:1164
float AsyncIOBandwidthLimit
Definition: LA_Core_classes.hpp:451
unsigned char UnknownData00[0x10]
Definition: LA_Core_classes.hpp:501
struct FVector GetVectorValue(float F, int LastExtreme)
Definition: LA_Basic.hpp:157
T * CreateDefaultObject()
Definition: LA_Core_classes.hpp:1247
float STATIC_MultiplyEqual_FloatFloat(float B, float *A)
int STATIC_FFloor(float A)
unsigned char UnknownData01[0xC]
Definition: LA_Core_classes.hpp:1077
struct FString STATIC_Locs(const struct FString &S)
void Enable(const struct FName &ProbeFunc)
bool STATIC_OrOr_BoolBool(bool A, bool B)
class UObject * Linker
Definition: LA_Core_classes.hpp:26
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:593
void STATIC_JoinArray(TArray< struct FString > StringArray, const struct FString &delim, bool bIgnoreBlanks, struct FString *out_Result)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:889
struct FVector STATIC_QuatRotateVector(const struct FQuat &A, const struct FVector &B)
float STATIC_Atan2(float A, float B)
unsigned char UnknownData00[0xA0]
Definition: LA_Core_classes.hpp:483
Definition: LA_Core_classes.hpp:743
struct FString Description
Definition: LA_Core_classes.hpp:1032
int STATIC_MultiplyEqual_IntFloat(float B, int *A)
struct FRotator STATIC_OrthoRotation(const struct FVector &X, const struct FVector &Y, const struct FVector &Z)
unsigned char UnknownData00[0x4]
Definition: LA_Core_classes.hpp:958
Definition: LA_Core_classes.hpp:778
float STATIC_Abs(float A)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:853
struct FQuat STATIC_QuatInvert(const struct FQuat &A)
float ByteToFloat(unsigned char inputByte, bool bSigned)
bool STATIC_EqualEqual_NameName(const struct FName &A, const struct FName &B)
Definition: LA_Core_classes.hpp:426
TArray< struct FString > FormatDescription
Definition: LA_Core_classes.hpp:1076
TArray< struct FString > STATIC_SplitString(const struct FString &Source, const struct FString &Delimiter, bool bCullEmpty)
unsigned char UnknownData00[0x8]
Definition: LA_Core_classes.hpp:869
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:835
struct FVector STATIC_Subtract_VectorVector(const struct FVector &A, const struct FVector &B)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:1208
bool STATIC_LessEqual_IntInt(int A, int B)
struct FString STATIC_GetScriptTrace()
float STATIC_Divide_FloatFloat(float A, float B)
struct FVector STATIC_GetRotatorAxis(const struct FRotator &A, int Axis)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:906
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:765
struct FVector STATIC_MultiplyEqual_VectorFloat(float B, struct FVector *A)
bool STATIC_GetPerObjectConfigSections(class UClass *SearchClass, class UObject *ObjectOuter, int MaxResults, TArray< struct FString > *out_SectionNames)
int STATIC_Xor_IntInt(int A, int B)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:924
unsigned char STATIC_AddEqual_ByteByte(unsigned char B, unsigned char *A)
void STATIC_ImportJSON(const struct FString &PropertyName, struct FString *JSON)
unsigned char STATIC_DivideEqual_ByteByte(unsigned char B, unsigned char *A)
uint32_t FunctionFlags
Definition: LA_Core_classes.hpp:702
Definition: LA_Core_classes.hpp:1009
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:1014
struct FVector2D STATIC_Multiply_Vector2DFloat(const struct FVector2D &A, float B)
void STATIC_GetAngularDegreesFromRadians(struct FVector2D *OutFOV)
Definition: LA_Core_classes.hpp:570
Definition: LA_Core_classes.hpp:699
int STATIC_Complement_PreInt(int A)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:1118
float STATIC_Cos(float A)
float STATIC_AddEqual_FloatFloat(float B, float *A)
TArray< struct FString > ReleaseCookedPaths
Definition: LA_Core_classes.hpp:456
unsigned char UnknownData00[0xD4]
Definition: LA_Core_classes.hpp:1206
float STATIC_Sqrt(float A)
float GetFloatValue(float F)
unsigned char FloatToByte(float inputFloat, bool bSigned)
struct FString STATIC_PathName(class UObject *CheckObject)
void EndState(const struct FName &NextStateName)
int STATIC_DivideEqual_IntFloat(float B, int *A)
void STATIC_ScriptTrace()
int MaxOverallCacheSize
Definition: LA_Core_classes.hpp:449
struct FVector2D STATIC_vect2d(float InX, float InY)
unsigned char STATIC_AddAdd_PreByte(unsigned char *A)
unsigned char UnknownData01[0x10]
Definition: LA_Core_classes.hpp:710
TArray< struct FString > CutdownPaths
Definition: LA_Core_classes.hpp:460
struct FPointer LinkerIndex
Definition: LA_Core_classes.hpp:27
Definition: LA_Core_classes.hpp:796
int GetBuildChangelistNumber()
Definition: LA_Core_structs.hpp:369
Definition: LA_Core_structs.hpp:342
float STATIC_RSize(const struct FRotator &R)
Definition: LA_AkAudio_classes.hpp:11
unsigned long ShowErrorCount
Definition: LA_Core_classes.hpp:1167
uint16_t RepOffset
Definition: LA_Core_classes.hpp:706
struct FVector STATIC_LessLess_VectorRotator(const struct FVector &A, const struct FRotator &B)
struct FVector STATIC_TransformNormal(const struct FMatrix &TM, const struct FVector &A)
bool STATIC_EqualEqual_InterfaceInterface(const TScriptInterface< class UInterface > &A, const TScriptInterface< class UInterface > &B)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:1140
struct FQuat STATIC_QuatFromAxisAndAngle(const struct FVector &Axis, float Angle)
struct FVector STATIC_MatrixGetOrigin(const struct FMatrix &TM)
unsigned char UnknownData00[0xC4]
Definition: LA_Core_classes.hpp:591
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:485
int STATIC_SubtractSubtract_PreInt(int *A)
struct FRotator STATIC_Multiply_FloatRotator(float A, const struct FRotator &B)
float STATIC_Subtract_FloatFloat(float A, float B)
struct FName STATIC_GetEnum(class UObject *E, int I)
TArray< struct FString > ValidGameNames
Definition: LA_Core_classes.hpp:1039
struct FString HelpWebLink
Definition: LA_Core_classes.hpp:1160
float STATIC_Exp(float A)
void STATIC_ProfNodeSetTimeThresholdSeconds(float Threshold)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:996
struct FString STATIC_ParseLocalizedPropertyPath(const struct FString &PathName)
struct FString HelpDescription
Definition: LA_Core_classes.hpp:1158
unsigned long bCanBeBaked
Definition: LA_Core_classes.hpp:1137
bool STATIC_Greater_StrStr(const struct FString &A, const struct FString &B)
Definition: LA_Core_classes.hpp:19
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:748
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:413
float PointDistToLine(const struct FVector &Point, const struct FVector &Line, const struct FVector &Origin, struct FVector *OutClosestPoint)
float STATIC_FInterpConstantTo(float Current, float Target, float DeltaTime, float InterpSpeed)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:1263
struct FVector2D STATIC_EvalInterpCurveVector2D(float InVal, struct FInterpCurveVector2D *Vector2DCurve)
struct FString STATIC_GetLanguage()
std::string GetFullName() const
float STATIC_FPctByRange(float Value, float InMin, float InMax)
class UObject * STATIC_FindObject(const struct FString &ObjectName, class UClass *ObjectClass)
void PopState(bool bPopAll)
struct FString HelpUsage
Definition: LA_Core_classes.hpp:1159
Definition: LA_Core_classes.hpp:1111
void PushState(const struct FName &NewState, const struct FName &NewLabel)
struct FName GetStateName()
bool STATIC_GetAngularDistance(const struct FVector &Direction, const struct FVector &AxisX, const struct FVector &AxisY, const struct FVector &AxisZ, struct FVector2D *OutAngularDist)
Definition: LA_Core_classes.hpp:444
unsigned char STATIC_SubtractEqual_ByteByte(unsigned char B, unsigned char *A)
struct FQuat STATIC_Add_QuatQuat(const struct FQuat &A, const struct FQuat &B)
float STATIC_FInterpEaseIn(float A, float B, float Alpha, float Exp)
Definition: LA_Core_classes.hpp:1155
Definition: LA_Core_structs.hpp:377
struct FRotator STATIC_QuatToRotator(const struct FQuat &A)
Definition: LA_Core_structs.hpp:217
struct FVector STATIC_InverseTransformVector(const struct FMatrix &TM, const struct FVector &A)
bool STATIC_LessEqual_FloatFloat(float A, float B)
struct FMatrix STATIC_Multiply_MatrixMatrix(const struct FMatrix &A, const struct FMatrix &B)
struct FRotator STATIC_DivideEqual_RotatorFloat(float B, struct FRotator *A)
unsigned char UnknownData00[0x40]
Definition: LA_Core_classes.hpp:728
struct FVector STATIC_PointProjectToPlane(const struct FVector &Point, const struct FVector &A, const struct FVector &B, const struct FVector &C)
struct FMatrix STATIC_MakeRotationTranslationMatrix(const struct FVector &Translation, const struct FRotator &Rotation)
struct FString STATIC_Localize(const struct FString &SectionName, const struct FString &KeyName, const struct FString &PackageName)
struct FPointer VfTable_FCurveEdInterface
Definition: LA_Core_classes.hpp:1136
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:628
uint16_t * ParmsSize
Definition: LA_Core_classes.hpp:708
bool IsInState(const struct FName &TestState, bool bTestStateStack)
Definition: LA_Core_classes.hpp:624
class UClass * TemplateOwnerClass
Definition: LA_Core_classes.hpp:1095
Definition: LA_Core_classes.hpp:848
unsigned char UnknownData00[0xB0]
Definition: LA_Core_classes.hpp:1242
struct FVector STATIC_VLerp(const struct FVector &A, const struct FVector &B, float Alpha)
bool STATIC_NotEqual_RotatorRotator(const struct FRotator &A, const struct FRotator &B)
struct FVector STATIC_VInterpTo(const struct FVector &Current, const struct FVector &Target, float DeltaTime, float InterpSpeed)
int STATIC_Clamp(int V, int A, int B)
float STATIC_RDiff(const struct FRotator &A, const struct FRotator &B)
unsigned char STATIC_SubtractSubtract_Byte(unsigned char *A)
class UField * Children
Definition: LA_Core_classes.hpp:664
struct FString STATIC_At_StrStr(const struct FString &A, const struct FString &B)
struct FString STATIC_Mid(const struct FString &S, int I, int J)
int STATIC_Or_IntInt(int A, int B)
class UField * SuperField
Definition: LA_Core_classes.hpp:663
Definition: LA_Core_structs.hpp:245
float STATIC_VSizeSq(const struct FVector &A)
class UObject * Outer
Definition: LA_Core_classes.hpp:30
struct FString STATIC_Caps(const struct FString &S)
Definition: LA_Core_classes.hpp:534
class UClass * ContextClass
Definition: LA_Core_classes.hpp:1031
struct FMatrix STATIC_MakeRotationMatrix(const struct FRotator &Rotation)
bool STATIC_ClassIsChildOf(class UClass *TestClass, class UClass *ParentClass)
bool STATIC_LessEqual_StrStr(const struct FString &A, const struct FString &B)
Definition: LA_Core_classes.hpp:1221
struct FVector STATIC_TransformVector(const struct FMatrix &TM, const struct FVector &A)
float STATIC_FInterpEaseOut(float A, float B, float Alpha, float Exp)
struct FVector STATIC_GreaterGreater_VectorRotator(const struct FVector &A, const struct FRotator &B)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:503
unsigned char UnknownData00[0x8]
Definition: LA_Core_classes.hpp:781
float STATIC_Lerp(float A, float B, float Alpha)
static TArray< UObject * > * GObjects
Definition: LA_Core_classes.hpp:22
Definition: LA_Basic.hpp:22
float STATIC_UnwindHeading(float A)
bool STATIC_EqualEqual_IntInt(int A, int B)
void STATIC_DebugBreak(int UserFlags, TEnumAsByte< EDebugBreakType > DebuggerType)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:871
struct FRotator STATIC_RotRand(bool bRoll)
float STATIC_Dot_VectorVector(const struct FVector &A, const struct FVector &B)
Definition: LA_Core_classes.hpp:516
TArray< struct FString > FormatExtension
Definition: LA_Core_classes.hpp:1075
struct FGuid STATIC_GetGuidFromString(struct FString *InGuidString)
float STATIC_VSize(const struct FVector &A)
bool STATIC_IsZero(const struct FVector &A)
void STATIC_InvalidateGuid(struct FGuid *InGuid)
struct FString STATIC_ToHex(int A)
float PointDistToSegment(const struct FVector &Point, const struct FVector &StartPoint, const struct FVector &EndPoint, struct FVector *OutClosestPoint)
struct FRotator STATIC_RInterpTo(const struct FRotator &Current, const struct FRotator &Target, float DeltaTime, float InterpSpeed, bool bConstantInterpSpeed)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:1188
static UClass * FindClass(const std::string &name)
Definition: LA_Core_classes.hpp:72
struct FVector STATIC_Add_VectorVector(const struct FVector &A, const struct FVector &B)
int STATIC_Max(int A, int B)
Definition: LA_Core_classes.hpp:1092
TArray< struct FDestinationLocObject > DestinationLocObjectInfoList
Definition: LA_Core_classes.hpp:537
int STATIC_LessLess_IntInt(int A, int B)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:646
bool IsChildState(const struct FName &TestState, const struct FName &TestParentState)
class UObject * STATIC_DynamicLoadObject(const struct FString &ObjectName, class UClass *ObjectClass, bool MayFail)
Definition: LA_Core_classes.hpp:641
struct FVector STATIC_Divide_VectorFloat(const struct FVector &A, float B)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:85
struct FString TimeStamp()
struct FVector STATIC_DivideEqual_VectorFloat(float B, struct FVector *A)
unsigned long IsEditor
Definition: LA_Core_classes.hpp:1165
struct FVector2D STATIC_MultiplyEqual_Vector2DFloat(float B, struct FVector2D *A)
unsigned char UnknownData00[0x8]
Definition: LA_Core_classes.hpp:887
Definition: LA_Core_structs.hpp:315
float STATIC_Asin(float A)
struct FVector STATIC_Normal(const struct FVector &A)
float PointDistToPlane(const struct FVector &Point, const struct FRotator &Orientation, const struct FVector &Origin, struct FVector *out_ClosestPoint)
struct FRotator STATIC_RLerp(const struct FRotator &A, const struct FRotator &B, float Alpha, bool bShortestPath)
std::string GetName() const
Definition: LA_Basic.hpp:212
Definition: LA_Core_classes.hpp:813
float STATIC_FMax(float A, float B)
unsigned long bCreateNew
Definition: LA_Core_classes.hpp:1034
Definition: LA_Core_classes.hpp:973
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:1079
Definition: LA_Core_classes.hpp:919
int STATIC_And_IntInt(int A, int B)
struct FVector2D STATIC_DivideEqual_Vector2DFloat(float B, struct FVector2D *A)
struct FVector TransformVectorByRotation(const struct FRotator &SourceRotation, const struct FVector &SourceVector, bool bInverse)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:611
struct FString STATIC_GetRightMost(const struct FString &Text)
struct FVector STATIC_InverseTransformNormal(const struct FMatrix &TM, const struct FVector &A)
bool STATIC_EqualEqual_BoolBool(bool A, bool B)
float STATIC_Square(float A)
bool STATIC_Not_PreBool(bool A)
bool STATIC_ClockwiseFrom_IntInt(int A, int B)
unsigned char UnknownData01[0xAC]
Definition: LA_Core_classes.hpp:1244
void GetSystemTime(int *Year, int *Month, int *DayOfWeek, int *Day, int *Hour, int *Min, int *Sec, int *MSec)
static UClass * StaticClass()
Definition: LA_Core_classes.hpp:668
struct FColor STATIC_Add_ColorColor(const struct FColor &A, const struct FColor &B)
Definition: LA_Core_classes.hpp:1184
class UClass * SupportedClass
Definition: LA_Core_classes.hpp:1030
bool STATIC_ComplementEqual_StrStr(const struct FString &A, const struct FString &B)
float STATIC_FInterpEaseInOut(float A, float B, float Alpha, float Exp)
bool STATIC_Greater_FloatFloat(float A, float B)
struct FString STATIC_Repl(const struct FString &Src, const struct FString &Match, const struct FString &With, bool bCaseSensitive)
bool STATIC_EqualEqual_FloatFloat(float A, float B)
void STATIC_GetUnAxes(const struct FRotator &A, struct FVector *X, struct FVector *Y, struct FVector *Z)
struct FRotator STATIC_Normalize(const struct FRotator &Rot)
int PackageSizeSoftLimit
Definition: LA_Core_classes.hpp:450
TArray< struct FString > Extensions
Definition: LA_Core_classes.hpp:462
float STATIC_VSizeSq2D(const struct FVector &A)
struct FVector STATIC_Normal2D(const struct FVector &A)
unsigned char UnknownData00[0x10]
Definition: LA_Core_classes.hpp:994
Definition: LA_Core_classes.hpp:1133
bool STATIC_Less_FloatFloat(float A, float B)
TArray< struct FString > Paths
Definition: LA_Core_classes.hpp:455
struct FVector STATIC_Subtract_PreVector(const struct FVector &A)
Definition: LA_Core_classes.hpp:725
int MaxStaleCacheSize
Definition: LA_Core_classes.hpp:448
unsigned char UnknownData00[0x8]
Definition: LA_Core_classes.hpp:746
float STATIC_QuatDot(const struct FQuat &A, const struct FQuat &B)
unsigned char UnknownData00[0x30]
Definition: LA_Core_classes.hpp:411
float STATIC_DivideEqual_FloatFloat(float B, float *A)
struct FName TemplateName
Definition: LA_Core_classes.hpp:1096
bool STATIC_GreaterEqual_StrStr(const struct FString &A, const struct FString &B)
void GotoState(const struct FName &NewState, const struct FName &Label, bool bForceEvents, bool bKeepStack)
float STATIC_VSize2D(const struct FVector &A)
Definition: LA_Basic.hpp:109
int STATIC_Min(int A, int B)