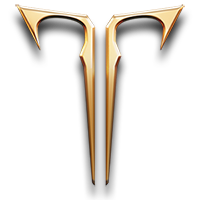 |
Lost Ark SDK
1.148.153.0
|
Definition: LA_Core_classes.hpp:1239
EASType
Definition: LA_GFxUI_structs.hpp:32
Definition: LA_Engine_classes.hpp:10673
class UTexture * Texture
Definition: LA_GFxUI_structs.hpp:124
float Rotation
Definition: LA_GFxUI_structs.hpp:159
unsigned long Visible
Definition: LA_GFxUI_structs.hpp:166
int m_statid
Definition: LA_GFxUI_structs.hpp:103
int I
Definition: LA_GFxUI_structs.hpp:114
int Index
Definition: LA_GFxUI_structs.hpp:190
Definition: LA_GFxUI_structs.hpp:108
int lastIndex
Definition: LA_GFxUI_structs.hpp:191
unsigned long hasYScale
Definition: LA_GFxUI_structs.hpp:174
unsigned long hasAlpha
Definition: LA_GFxUI_structs.hpp:176
Definition: LA_Core_structs.hpp:253
float Alpha
Definition: LA_GFxUI_structs.hpp:165
Definition: LA_GFxUI_classes.hpp:225
Definition: LA_Engine_classes.hpp:30547
float ZScale
Definition: LA_GFxUI_structs.hpp:164
Definition: LA_Basic.hpp:69
float X
Definition: LA_GFxUI_structs.hpp:156
unsigned long B
Definition: LA_GFxUI_structs.hpp:112
@ FlashTextureScale_NextLow
float YRotation
Definition: LA_GFxUI_structs.hpp:161
struct FString S
Definition: LA_GFxUI_structs.hpp:115
class UObject * m_object
Definition: LA_GFxUI_structs.hpp:101
float N
Definition: LA_GFxUI_structs.hpp:113
class UGFxObject * O
Definition: LA_GFxUI_structs.hpp:116
unsigned long hasZScale
Definition: LA_GFxUI_structs.hpp:175
unsigned long hasXScale
Definition: LA_GFxUI_structs.hpp:173
EGFxAlign
Definition: LA_GFxUI_structs.hpp:47
float Z
Definition: LA_GFxUI_structs.hpp:158
int mouseIndex
Definition: LA_GFxUI_structs.hpp:188
Definition: LA_GFxUI_structs.hpp:129
TEnumAsByte< EASType > Type
Definition: LA_GFxUI_structs.hpp:110
Definition: LA_Basic.hpp:157
unsigned char UnknownData00[0x3]
Definition: LA_GFxUI_structs.hpp:111
float Y
Definition: LA_GFxUI_structs.hpp:157
int Data
Definition: LA_GFxUI_structs.hpp:187
EGFxRenderTextureMode
Definition: LA_GFxUI_structs.hpp:83
class UGFxObject * _this
Definition: LA_GFxUI_structs.hpp:184
int Button
Definition: LA_GFxUI_structs.hpp:189
unsigned long hasRotation
Definition: LA_GFxUI_structs.hpp:170
Definition: LA_AkAudio_classes.hpp:11
float XScale
Definition: LA_GFxUI_structs.hpp:162
Definition: LA_GFxUI_structs.hpp:99
Definition: LA_GFxUI_structs.hpp:182
struct FString Resource
Definition: LA_GFxUI_structs.hpp:123
@ FlashTextureScale_Mult4
Definition: LA_Core_classes.hpp:19
unsigned long hasY
Definition: LA_GFxUI_structs.hpp:168
EFlashTextureRescale
Definition: LA_GFxUI_structs.hpp:20
unsigned long hasX
Definition: LA_GFxUI_structs.hpp:167
struct FString ThemeClassName
Definition: LA_GFxUI_structs.hpp:133
unsigned long hasVisible
Definition: LA_GFxUI_structs.hpp:177
Definition: LA_GFxUI_structs.hpp:154
EGFxScaleMode
Definition: LA_GFxUI_structs.hpp:63
unsigned long hasZ
Definition: LA_GFxUI_structs.hpp:169
Definition: LA_GFxUI_structs.hpp:121
EGFxTimingMode
Definition: LA_GFxUI_structs.hpp:74
unsigned long hasXRotation
Definition: LA_GFxUI_structs.hpp:171
class UGFxObject * Target
Definition: LA_GFxUI_structs.hpp:185
class UUISoundTheme * Theme
Definition: LA_GFxUI_structs.hpp:132
float YScale
Definition: LA_GFxUI_structs.hpp:163
struct FString Type
Definition: LA_GFxUI_structs.hpp:186
unsigned long hasYRotation
Definition: LA_GFxUI_structs.hpp:172
struct FName ThemeName
Definition: LA_GFxUI_structs.hpp:131
int m_count
Definition: LA_GFxUI_structs.hpp:102
float XRotation
Definition: LA_GFxUI_structs.hpp:160