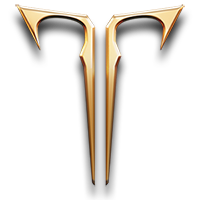 |
Lost Ark SDK
1.148.153.0
|
Go to the documentation of this file.
6 #pragma pack(push, 0x4)
27 static UClass* ptr =
nullptr;
44 static UClass* ptr =
nullptr;
65 static UClass* ptr =
nullptr;
139 static UClass* ptr =
nullptr;
203 void SetViewport(
int X,
int Y,
int Width,
int Height);
214 void Close(
bool Unload);
219 bool Start(
bool StartPaused);
235 static UClass* ptr =
nullptr;
333 static UClass* ptr =
nullptr;
363 static UClass* ptr =
nullptr;
393 static UClass* ptr =
nullptr;
410 static UClass* ptr =
nullptr;
429 static UClass* ptr =
nullptr;
450 static UClass* ptr =
nullptr;
472 static UClass* ptr =
nullptr;
504 static UClass* ptr =
nullptr;
525 static UClass* ptr =
nullptr;
544 static UClass* ptr =
nullptr;
566 static UClass* ptr =
nullptr;
583 static UClass* ptr =
nullptr;
struct FString Variable
Definition: LA_GFxUI_classes.hpp:446
Definition: LA_Core_classes.hpp:1239
Definition: LA_Engine_classes.hpp:28280
int LocalPlayerOwnerIndex
Definition: LA_GFxUI_classes.hpp:120
TEnumAsByte< EFlashTextureRescale > TextureRescale
Definition: LA_GFxUI_classes.hpp:383
Definition: LA_Engine_classes.hpp:10673
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:408
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:542
void SetElementPosition(int Index, float X, float Y)
struct FASDisplayInfo GetDisplayInfo()
int ActionScriptInt(const struct FString &method)
bool IsFloat(const struct FString &Member)
void ActionScriptSetFunction(const struct FString &Member)
bool Start(bool StartPaused)
Definition: LA_GFxUI_classes.hpp:485
void GotoAndPlay(const struct FString &frame)
unsigned long bListenExternalInterfaceCall
Definition: LA_GFxUI_classes.hpp:231
unsigned long bCloseOnLevelChange
Definition: LA_GFxUI_classes.hpp:104
Definition: LA_GFxUI_classes.hpp:463
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:391
bool IsValidLevelSequenceObject()
Definition: LA_Engine_classes.hpp:25968
class UGFxObject * GetVariableObject(const struct FString &Path, class UClass *Type)
unsigned long bForceFullViewport
Definition: LA_GFxUI_classes.hpp:106
unsigned long bWidgetsInitializedThisFrame
Definition: LA_GFxUI_classes.hpp:96
struct FMatrix GetElementDisplayMatrix(int Index)
class UGFxMoviePlayer * MoviePlayer
Definition: LA_GFxUI_classes.hpp:490
class ULocalPlayer * GetLP()
unsigned long bIsSplitscreenLayoutModified
Definition: LA_GFxUI_classes.hpp:111
Definition: LA_GFxUI_structs.hpp:108
struct FQWord ImportTimeStamp
Definition: LA_GFxUI_classes.hpp:389
void SetText(const struct FString &Text, class UTranslationContext *InContext)
unsigned long bDisplayWithHudOff
Definition: LA_GFxUI_classes.hpp:94
void SetFunction(const struct FString &Member, class UObject *context, const struct FName &fname)
unsigned long bShowHardwareMouseCursor
Definition: LA_GFxUI_classes.hpp:112
class USwfMovie * MovieInfo
Definition: LA_GFxUI_classes.hpp:92
void SetElementMemberObject(int Index, const struct FString &Member, class UGFxObject *val)
Definition: LA_GFxUI_classes.hpp:225
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:25
bool SetVariableFloatArray(const struct FString &Path, int Index, TArray< float > Arg)
struct FString PathInfo
Definition: LA_GFxUI_classes.hpp:229
class UGFxMoviePlayer * Movie
Definition: LA_GFxUI_classes.hpp:466
bool IsString(const struct FString &Member)
struct FASValue GetElementMember(int Index, const struct FString &Member)
Definition: LA_GFxUI_classes.hpp:38
void PlaySoundFromTheme(const struct FName &EventName, const struct FName &SoundThemeName)
class UGFxMoviePlayer * Movie
Definition: LA_GFxUI_classes.hpp:539
void SetElementDisplayMatrix(int Index, const struct FMatrix &M)
bool GetVariableIntArray(const struct FString &Path, int Index, TArray< int > *Arg)
void ApplyPriorityBlurEffect(bool bRemoveEffect)
bool WidgetUnloaded(const struct FName &WidgetName, const struct FName &WidgetPath, class UGFxObject *Widget)
int SplitscreenLayoutYAdjust
Definition: LA_GFxUI_classes.hpp:133
void SetTimingMode(TEnumAsByte< EGFxTimingMode > Mode)
void SetVariableNumber(const struct FString &Path, float F)
unsigned long bEnableGammaCorrection
Definition: LA_GFxUI_classes.hpp:494
TArray< class UGFxObject * > DebugArray
Definition: LA_GFxUI_classes.hpp:134
int RTTextures
Definition: LA_GFxUI_classes.hpp:387
unsigned long bEnableGammaCorrection
Definition: LA_GFxUI_classes.hpp:95
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:564
Definition: LA_Basic.hpp:69
TEnumAsByte< EGFxRenderTextureMode > RenderTextureMode
Definition: LA_GFxUI_classes.hpp:496
unsigned long bMovieIsOpen
Definition: LA_GFxUI_classes.hpp:93
unsigned long bAllowFocus
Definition: LA_GFxUI_classes.hpp:99
struct FScriptDelegate __OnPostAdvance__Delegate
Definition: LA_GFxUI_classes.hpp:135
int RTVideoTextures
Definition: LA_GFxUI_classes.hpp:388
TArray< struct FGFxWidgetBinding > SubWidgetBindings
Definition: LA_GFxUI_classes.hpp:230
class UObject * ExternalInterface
Definition: LA_GFxUI_classes.hpp:121
unsigned long bAutoPlay
Definition: LA_GFxUI_classes.hpp:100
class UGFxObject * GetElementObjectEx(int Index, const struct FString &SourceFile, int SourceLine, class UClass *Type, const struct FString &ASClass)
struct FString FSCommand
Definition: LA_GFxUI_classes.hpp:561
void Set(const struct FString &Member, const struct FASValue &Arg)
void SetVariableObject(const struct FString &Path, class UGFxObject *Object)
void GotoAndStop(const struct FString &frame)
unsigned long bBlurLesserMovies
Definition: LA_GFxUI_classes.hpp:113
bool SetVariableArray(const struct FString &Path, int Index, TArray< struct FASValue > Arg)
Definition: LA_GFxUI_classes.hpp:577
unsigned long bUsesFontlib
Definition: LA_GFxUI_classes.hpp:377
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:233
void SetWidgetPathBinding(class UGFxObject *WidgetToBind, const struct FName &Path)
bool GetPosition(float *X, float *Y)
TArray< struct FName > CaptureKeys
Definition: LA_GFxUI_classes.hpp:521
unsigned char UnknownData00[0x3]
Definition: LA_GFxUI_classes.hpp:497
bool SetVariableStringArray(const struct FString &Path, int Index, TArray< struct FString > Arg)
void SetMovieCanReceiveFocus(bool bCanReceiveFocus)
Definition: LA_GFxUI_classes.hpp:374
void SetColorTransform(const struct FASColorTransform &cxform)
void ActionScriptVoid(const struct FString &Path)
unsigned char Priority
Definition: LA_GFxUI_classes.hpp:128
void AddCaptureKey(const struct FName &Key)
struct FString ActionScriptString(const struct FString &Path)
TArray< struct FSoundThemeBinding > SoundThemes
Definition: LA_GFxUI_classes.hpp:125
TArray< struct FName > CaptureKeys
Definition: LA_GFxUI_classes.hpp:122
void NotifyGameSessionEnded()
Definition: LA_Engine_classes.hpp:32179
bool SetExternalTextureEx(const struct FString &Resource, class UTexture *Texture, int targetWidth, int targetHeight, bool targetStretch)
void SetElementMemberString(int Index, const struct FString &Member, const struct FString &S)
void SetVariableBool(const struct FString &Path, bool B)
class UTextureRenderTarget2D * RenderTexture
Definition: LA_GFxUI_classes.hpp:498
void GetVisibleFrameRect(float *x0, float *y0, float *X1, float *Y1)
Definition: LA_Engine_classes.hpp:8954
void NotifyPlayerAdded(int PlayerIndex, class ULocalPlayer *AddedPlayer)
unsigned long bStartPaused
Definition: LA_GFxUI_classes.hpp:493
void SetObject(const struct FString &Member, class UGFxObject *val)
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:502
void SetAlignment(TEnumAsByte< EGFxAlign > A)
unsigned long bUnload
Definition: LA_GFxUI_classes.hpp:425
unsigned char UnknownData00[0x48]
Definition: LA_GFxUI_classes.hpp:89
Definition: LA_Engine_classes.hpp:10914
struct FPointer pFocusIgnoreKeys
Definition: LA_GFxUI_classes.hpp:88
Definition: LA_Core_structs.hpp:359
TArray< class UObject * > UserReferences
Definition: LA_GFxUI_classes.hpp:359
struct FMatrix GetDisplayMatrix()
class APlayerController * GetPC()
void ActionScriptVoid(const struct FString &method)
Definition: LA_GFxUI_classes.hpp:57
Definition: LA_GFxUI_classes.hpp:517
void SetElementDisplayInfo(int Index, const struct FASDisplayInfo &D)
void SetVariable(const struct FString &Path, const struct FASValue &Arg)
float GetVariableNumber(const struct FString &Path)
void SetElementString(int Index, const struct FString &S)
TArray< struct FASValue > Arguments
Definition: LA_GFxUI_classes.hpp:468
int RefCount
Definition: LA_GFxUI_classes.hpp:23
Definition: LA_GFxUI_classes.hpp:557
void ConsoleCommand(const struct FString &Command)
class UTextureRenderTarget2D * RenderTexture
Definition: LA_GFxUI_classes.hpp:119
int Value[0xC]
Definition: LA_GFxUI_classes.hpp:228
void SetDisplayMatrix(const struct FMatrix &M)
class UGFxObject * SplitscreenLayoutObject
Definition: LA_GFxUI_classes.hpp:132
Definition: LA_Engine_classes.hpp:10828
void ConditionalClearPause()
struct FPointer pCaptureKeys
Definition: LA_GFxUI_classes.hpp:87
void SetVisible(bool Visible)
void FlushPlayerInput(bool capturekeysonly)
unsigned long bIgnoreBlurEffect
Definition: LA_GFxUI_classes.hpp:118
void ActionScriptSetFunction(class UGFxObject *Object, const struct FString &Member)
class UGFxMoviePlayer * Movie
Definition: LA_GFxUI_classes.hpp:520
int GetInt(const struct FString &Member)
struct FPointer pMovie
Definition: LA_GFxUI_classes.hpp:86
unsigned long bTakeFocus
Definition: LA_GFxUI_classes.hpp:491
int NextASUObject
Definition: LA_GFxUI_classes.hpp:91
void SetPause(bool bPausePlayback)
float ActionScriptFloat(const struct FString &Path)
unsigned long bPauseGameWhileActive
Definition: LA_GFxUI_classes.hpp:101
struct FASValue Get(const struct FString &Member)
void ApplyPriorityVisibilityEffect(bool bRemoveEffect)
TEnumAsByte< EGFxRenderTextureMode > RenderTextureMode
Definition: LA_GFxUI_classes.hpp:127
void AddFocusIgnoreKey(const struct FName &Key)
class UGFxObject * GetElementObject(int Index, const struct FString &SourceFile, int SourceLine, class UClass *Type)
void SetVariableString(const struct FString &Path, const struct FString &S)
Definition: LA_GFxUI_classes.hpp:442
void SetViewScaleMode(TEnumAsByte< EGFxScaleMode > SM)
Definition: LA_Basic.hpp:157
unsigned long bPackTextures
Definition: LA_GFxUI_classes.hpp:379
Definition: LA_GFxUI_classes.hpp:404
bool GetVariableFloatArray(const struct FString &Path, int Index, TArray< float > *Arg)
bool WidgetInitialized(const struct FName &WidgetName, const struct FName &WidgetPath, class UGFxObject *Widget)
unsigned long bCaptureInput
Definition: LA_GFxUI_classes.hpp:108
void SetString(const struct FString &Member, const struct FString &S, class UTranslationContext *InContext)
class UGFxMoviePlayer * GetFocusMovie(int ControllerId)
TArray< struct FGFxWidgetBinding > WidgetBindings
Definition: LA_GFxUI_classes.hpp:130
bool IsInt(const struct FString &Member)
Definition: LA_GFxUI_classes.hpp:536
void OnFocusLost(int LocalPlayerIndex)
class UGFxObject * GetElementMemberObject(int Index, const struct FString &Member, const struct FString &SourceFile, int SourceLine, class UClass *Type)
bool WidgetInitialized(const struct FName &WidgetName, const struct FName &WidgetPath, class UGFxObject *Widget)
class UGFxObject * CreateObject(const struct FString &ASClass, class UClass *Type, TArray< struct FASValue > args)
struct FString GetElementMemberString(int Index, const struct FString &Member)
Definition: LA_Basic.hpp:268
float ActionScriptFloat(const struct FString &method)
void SetFloat(const struct FString &Member, float F)
struct FASValue _Invoke(const struct FString &method, TArray< struct FASValue > args)
void SetElement(int Index, const struct FASValue &Arg)
struct FString SourceFile
Definition: LA_GFxUI_classes.hpp:381
bool SetVariableIntArray(const struct FString &Path, int Index, TArray< int > Arg)
class UGFxFSCmdHandler_Kismet * Handler
Definition: LA_GFxUI_classes.hpp:562
void OnPostAdvance(float DeltaTime)
TArray< struct FName > FocusIgnoreKeys
Definition: LA_GFxUI_classes.hpp:123
float GetFloat(const struct FString &Member)
struct FString GetElementString(int Index)
Definition: LA_Engine_classes.hpp:6076
void SetPriority(unsigned char NewPriority)
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:581
unsigned char UnknownData02[0x1]
Definition: LA_GFxUI_classes.hpp:129
struct FString Variable
Definition: LA_GFxUI_classes.hpp:540
bool FilterButtonInput(int ControllerId, const struct FName &ButtonName, TEnumAsByte< EInputEvent > InputEvent)
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:523
void OnFocusGained(int LocalPlayerIndex)
void SetElementFloat(int Index, float F)
void Init(class ULocalPlayer *LocPlay)
void ClearFocusIgnoreKeys()
bool FSCommand(class UGFxMoviePlayer *Movie, class UGFxEvent_FSCommand *Event, const struct FString &Cmd, const struct FString &Arg)
unsigned long bIsPriorityHidden
Definition: LA_GFxUI_classes.hpp:116
bool SetExternalTexture(const struct FString &Resource, class UTexture *Texture)
Definition: LA_Core_structs.hpp:369
int ActionScriptInt(const struct FString &Path)
Definition: LA_AkAudio_classes.hpp:11
void SetPerspective3D(struct FMatrix *matPersp)
struct FString GetVariableString(const struct FString &Path)
class UGFxObject * GetElementMemberObjectEx(int Index, const struct FString &Member, const struct FString &SourceFile, int SourceLine, class UClass *Type, const struct FString &ASClass)
void SetElementMember(int Index, const struct FString &Member, const struct FASValue &Arg)
TEnumAsByte< EGFxTimingMode > TimingMode
Definition: LA_GFxUI_classes.hpp:126
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:448
void SetElementMemberBool(int Index, const struct FString &Member, bool B)
void SetBool(const struct FString &Member, bool B)
struct FString TextureFormat
Definition: LA_GFxUI_classes.hpp:385
void SetExternalInterface(class UObject *H)
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:42
Definition: LA_GFxUI_structs.hpp:182
int GetElementMemberInt(int Index, const struct FString &Member)
Definition: LA_Core_classes.hpp:19
struct FString GetString(const struct FString &Member)
TArray< class UObject * > References
Definition: LA_GFxUI_classes.hpp:358
bool FSCommand(class UGFxMoviePlayer *Movie, class UGFxEvent_FSCommand *Event, const struct FString &Cmd, const struct FString &Arg)
Definition: LA_GFxUI_classes.hpp:83
unsigned long bIgnoreMouseInput
Definition: LA_GFxUI_classes.hpp:110
struct FASValue GetElement(int Index)
class UGFxMoviePlayer * Movie
Definition: LA_GFxUI_classes.hpp:445
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:137
class UGFxObject * GetObject(const struct FString &Member, const struct FString &SourceFile, int SourceLine, class UClass *Type)
bool IsValidLevelSequenceObject()
bool GetVariableStringArray(const struct FString &Path, int Index, TArray< struct FString > *Arg)
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:470
void SetDisplayInfo(const struct FASDisplayInfo &D)
int GetVariableInt(const struct FString &Path)
struct FASValue _Invoke(const struct FString &Member, TArray< struct FASValue > args)
void SetElementColorTransform(int Index, const struct FASColorTransform &cxform)
Definition: LA_Core_structs.hpp:377
void SetElementMemberFloat(int Index, const struct FString &Member, float F)
struct FString STATIC_TranslateString(const struct FString &StringToTranslate, class UTranslationContext *InContext)
class UClass * MoviePlayerClass
Definition: LA_GFxUI_classes.hpp:489
void SetElementBool(int Index, bool B)
bool GetElementMemberBool(int Index, const struct FString &Member)
bool IsValidLevelSequenceObject()
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:361
struct FASDisplayInfo GetElementDisplayInfo(int Index)
unsigned long bDisplayWithHudOff
Definition: LA_GFxUI_classes.hpp:495
void SetDisplayMatrix3D(const struct FMatrix &M)
struct FPointer VfTable_FCallbackEventDevice
Definition: LA_GFxUI_classes.hpp:60
bool IsValidLevelSequenceObject()
Definition: LA_GFxUI_structs.hpp:154
void ApplyPriorityEffect(bool bRequestedBlurState, bool bRequestedHiddenState)
void GotoAndStopI(int frame)
float GetElementMemberFloat(int Index, const struct FString &Member)
struct FMatrix GetDisplayMatrix3D()
unsigned long bFakeMobileTouches
Definition: LA_GFxUI_classes.hpp:61
void SetElementMemberInt(int Index, const struct FString &Member, int I)
void SetViewport(int X, int Y, int Width, int Height)
unsigned long bForceSquarePacking
Definition: LA_GFxUI_classes.hpp:380
unsigned long bDisableWorldRendering
Definition: LA_GFxUI_classes.hpp:102
bool GetVariableArray(const struct FString &Path, int Index, TArray< struct FASValue > *Arg)
struct FASColorTransform GetColorTransform()
unsigned long bCaptureMouseInput
Definition: LA_GFxUI_classes.hpp:109
struct FString ActionScriptString(const struct FString &method)
void SetElementVisible(int Index, bool Visible)
void CloseAllMoviePlayers()
unsigned long bOnlyOwnerFocusable
Definition: LA_GFxUI_classes.hpp:105
unsigned long bAllowInput
Definition: LA_GFxUI_classes.hpp:98
TArray< struct FExternalTexture > ExternalTextures
Definition: LA_GFxUI_classes.hpp:124
Definition: LA_Engine_classes.hpp:9806
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:427
bool GetVariableBool(const struct FString &Path)
TArray< struct FString > ReferencedSwfs
Definition: LA_GFxUI_classes.hpp:357
class USwfMovie * Movie
Definition: LA_GFxUI_classes.hpp:488
void SetPosition(float X, float Y)
unsigned long bSetSRGBOnImportedTextures
Definition: LA_GFxUI_classes.hpp:378
class USwfMovie * Movie
Definition: LA_GFxUI_classes.hpp:560
void SetMovieCanReceiveInput(bool bCanReceiveInput)
Definition: LA_Basic.hpp:22
TArray< struct FName > CaptureKeys
Definition: LA_GFxUI_classes.hpp:499
struct FString SourceFileTimestamp
Definition: LA_GFxUI_classes.hpp:386
bool GetBool(const struct FString &Member)
void SetVariableInt(const struct FString &Path, int I)
Definition: LA_GFxUI_classes.hpp:353
static UClass * FindClass(const std::string &name)
Definition: LA_Core_classes.hpp:72
bool IsBool(const struct FString &Member)
void SetElementObject(int Index, class UGFxObject *val)
Definition: LA_GFxUI_classes.hpp:19
static UClass * StaticClass()
Definition: LA_GFxUI_classes.hpp:63
int GetElementInt(int Index)
unsigned long bDiscardNonOwnerInput
Definition: LA_GFxUI_classes.hpp:107
unsigned long bIgnoreVisibilityEffect
Definition: LA_GFxUI_classes.hpp:117
void SetElementInt(int Index, int I)
struct FASValue GetVariable(const struct FString &Path)
class UGameViewportClient * GetGameViewportClient()
void SetView3D(struct FMatrix *matView)
void SetInt(const struct FString &Member, int I)
bool IsObject(const struct FString &Member)
void UpdateSplitscreenLayout()
struct FString MethodName
Definition: LA_GFxUI_classes.hpp:467
unsigned long bLogUnhandedWidgetInitializations
Definition: LA_GFxUI_classes.hpp:97
float GetElementFloat(int Index)
void SetMovieInfo(class USwfMovie *Data)
bool IsValidLevelSequenceObject()
unsigned long bCaptureWorldRendering
Definition: LA_GFxUI_classes.hpp:103
unsigned long bHideLesserMovies
Definition: LA_GFxUI_classes.hpp:114
TArray< struct FName > FocusIgnoreKeys
Definition: LA_GFxUI_classes.hpp:500
TArray< unsigned char > RawData
Definition: LA_GFxUI_classes.hpp:356
TArray< struct FGCReference > GCReferences
Definition: LA_GFxUI_classes.hpp:22
class UGFxMoviePlayer * Movie
Definition: LA_GFxUI_classes.hpp:424
unsigned long bCaptureInput
Definition: LA_GFxUI_classes.hpp:492
int PackTextureSize
Definition: LA_GFxUI_classes.hpp:382
unsigned long bIsPriorityBlurred
Definition: LA_GFxUI_classes.hpp:115
void NotifySplitscreenLayoutChanged()
void ActionScriptSetFunctionOn(class UGFxObject *Target, const struct FString &Member)
unsigned char UnknownData00[0x3]
Definition: LA_GFxUI_classes.hpp:384
unsigned char UnknownData01[0x48]
Definition: LA_GFxUI_classes.hpp:90
void NotifyPlayerRemoved(int PlayerIndex, class ULocalPlayer *RemovedPlayer)
bool WidgetUnloaded(const struct FName &WidgetName, const struct FName &WidgetPath, class UGFxObject *Widget)
unsigned char UnknownData03[0x48]
Definition: LA_GFxUI_classes.hpp:131
bool GetElementBool(int Index)
class UGFxObject * CreateArray(class UClass *Type)
void GotoAndPlayI(int frame)
Definition: LA_GFxUI_classes.hpp:421
void PostAdvance(float DeltaTime)