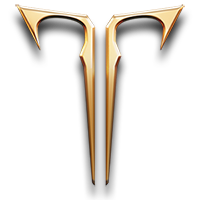 |
Lost Ark SDK
1.148.153.0
|
Go to the documentation of this file.
6 #pragma pack(push, 0x4)
30 static UClass* ptr =
nullptr;
48 static UClass* ptr =
nullptr;
79 static UClass* ptr =
nullptr;
87 void SetDesiredRotation(
const struct FRotator& TargetDesiredRotation,
bool InLockDesiredRotation,
bool InUnlockWhenReached,
float InterpolationTime);
124 static UClass* ptr =
nullptr;
146 void Tick(
float DeltaTime);
167 static UClass* ptr =
nullptr;
189 static UClass* ptr =
nullptr;
196 void ClientColorFade(
const struct FColor& FadeColor,
unsigned char FromAlpha,
unsigned char ToAlpha,
float FadeTime);
200 void ClientStopMovie(
float DelayInSeconds,
bool bAllowMovieToFinish,
bool bForceStopNonSkippable,
bool bForceStopLoadingMovie);
201 void ClientPlayMovie(
const struct FString& MovieName,
int InStartOfRenderingMovieFrame,
int InEndOfRenderingMovieFrame,
bool bRestrictPausing,
bool bPlayOnceFromStream,
bool bOnlyBackButtonSkipsMovie);
203 void STATIC_ShowLoadingMovie(
bool bShowMovie,
bool bPauseAfterHide,
float PauseDuration,
float KeepPlayingDuration,
bool bOverridePreviousDelays);
225 static UClass* ptr =
nullptr;
347 static UClass* ptr =
nullptr;
435 static UClass* ptr =
nullptr;
465 static UClass* ptr =
nullptr;
506 static UClass* ptr =
nullptr;
527 void Tick(
float DeltaTime);
553 static UClass* ptr =
nullptr;
579 static UClass* ptr =
nullptr;
604 static UClass* ptr =
nullptr;
628 static UClass* ptr =
nullptr;
653 static UClass* ptr =
nullptr;
675 static UClass* ptr =
nullptr;
697 static UClass* ptr =
nullptr;
722 static UClass* ptr =
nullptr;
780 static UClass* ptr =
nullptr;
821 static UClass* ptr =
nullptr;
862 static UClass* ptr =
nullptr;
882 void Tick(
float DeltaTime);
909 static UClass* ptr =
nullptr;
928 static UClass* ptr =
nullptr;
945 static UClass* ptr =
nullptr;
947 ptr =
UObject::FindClass(
"Class GameFramework.GameDestinationConnRenderingComponent");
1013 static UClass* ptr =
nullptr;
1051 static UClass* ptr =
nullptr;
1090 static UClass* ptr =
nullptr;
1123 static UClass* ptr =
nullptr;
1155 static UClass* ptr =
nullptr;
1198 static UClass* ptr =
nullptr;
1314 static UClass* ptr =
nullptr;
1379 static UClass* ptr =
nullptr;
1408 static UClass* ptr =
nullptr;
1454 static UClass* ptr =
nullptr;
1524 static UClass* ptr =
nullptr;
1580 static UClass* ptr =
nullptr;
1605 static UClass* ptr =
nullptr;
1607 ptr =
UObject::FindClass(
"Class GameFramework.NavMeshPath_BiasAgainstPolysWithinDistanceOfLocations");
1625 static UClass* ptr =
nullptr;
1644 static UClass* ptr =
nullptr;
1666 static UClass* ptr =
nullptr;
1689 static UClass* ptr =
nullptr;
1724 static UClass* ptr =
nullptr;
1726 ptr =
UObject::FindClass(
"Class GameFramework.SeqAct_GameCrowdPopulationManagerToggle");
1746 static UClass* ptr =
nullptr;
1767 static UClass* ptr =
nullptr;
1784 static UClass* ptr =
nullptr;
1802 static UClass* ptr =
nullptr;
1819 static UClass* ptr =
nullptr;
1839 static UClass* ptr =
nullptr;
1866 static UClass* ptr =
nullptr;
1886 static UClass* ptr =
nullptr;
1888 ptr =
UObject::FindClass(
"Class GameFramework.SeqEvent_CrowdAgentReachedDestination");
1906 static UClass* ptr =
nullptr;
1926 static UClass* ptr =
nullptr;
1952 static UClass* ptr =
nullptr;
1972 static UClass* ptr =
nullptr;
1994 static UClass* ptr =
nullptr;
2017 static UClass* ptr =
nullptr;
2037 static UClass* ptr =
nullptr;
2059 static UClass* ptr =
nullptr;
2084 static UClass* ptr =
nullptr;
2106 static UClass* ptr =
nullptr;
2129 static UClass* ptr =
nullptr;
2148 static UClass* ptr =
nullptr;
2226 static UClass* ptr =
nullptr;
2244 void SetFocusOnActor(
class AActor* FocusActor,
const struct FName& FocusBoneName,
const struct FVector2D& InterpSpeedRange,
const struct FVector2D& InFocusFOV,
float CameraFOV,
bool bAlwaysFocus,
bool bAdjustCamera,
bool bIgnoreTrace,
float FocusPitchOffsetDeg);
2245 void SetFocusOnLoc(
const struct FVector& FocusWorldLoc,
const struct FVector2D& InterpSpeedRange,
const struct FVector2D& InFocusFOV,
float CameraFOV,
bool bAlwaysFocus,
bool bAdjustCamera,
bool bIgnoreTrace,
float FocusPitchOffsetDeg);
2248 void BeginTurn(
int StartAngle,
int EndAngle,
float TimeSec,
float DelaySec,
bool bAlignTargetWhenFinished);
2279 static UClass* ptr =
nullptr;
2367 static UClass* ptr =
nullptr;
2402 static UClass* ptr =
nullptr;
2433 static UClass* ptr =
nullptr;
2450 void Tick(
float DeltaTime);
2480 static UClass* ptr =
nullptr;
2512 static UClass* ptr =
nullptr;
2534 static UClass* ptr =
nullptr;
2555 static UClass* ptr =
nullptr;
2573 static UClass* ptr =
nullptr;
2594 static UClass* ptr =
nullptr;
2613 static UClass* ptr =
nullptr;
2633 static UClass* ptr =
nullptr;
2652 static UClass* ptr =
nullptr;
2680 static UClass* ptr =
nullptr;
2719 static UClass* ptr =
nullptr;
2732 void Tick(
float DeltaTime);
2750 static UClass* ptr =
nullptr;
2767 static UClass* ptr =
nullptr;
2803 static UClass* ptr =
nullptr;
2821 static UClass* ptr =
nullptr;
2841 static UClass* ptr =
nullptr;
2864 static UClass* ptr =
nullptr;
2887 static UClass* ptr =
nullptr;
2907 static UClass* ptr =
nullptr;
2933 static UClass* ptr =
nullptr;
2963 static UClass* ptr =
nullptr;
2987 static UClass* ptr =
nullptr;
3012 static UClass* ptr =
nullptr;
3034 static UClass* ptr =
nullptr;
3055 static UClass* ptr =
nullptr;
3083 static UClass* ptr =
nullptr;
3125 static UClass* ptr =
nullptr;
3162 static UClass* ptr =
nullptr;
3198 static UClass* ptr =
nullptr;
3219 static UClass* ptr =
nullptr;
3242 static UClass* ptr =
nullptr;
3264 static UClass* ptr =
nullptr;
3281 static UClass* ptr =
nullptr;
3304 static UClass* ptr =
nullptr;
3325 static UClass* ptr =
nullptr;
3351 static UClass* ptr =
nullptr;
3375 static UClass* ptr =
nullptr;
float GetDesiredFOV(class APawn *ViewedPawn)
void Tick(float DeltaTime)
bool CalcCamera(float fDeltaTime, struct FVector *out_CamLoc, struct FRotator *out_CamRot, float *out_FOV)
float AuthoredGlobalScale
Definition: LA_GameFramework_classes.hpp:1902
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:577
unsigned long bShowGameHud
Definition: LA_GameFramework_classes.hpp:1169
Definition: LA_GameFramework_classes.hpp:2627
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2862
void SetCurrentDestination(class AGameCrowdDestination *NewDest)
struct FTextureUVs ButtonUVs[0x2]
Definition: LA_GameFramework_classes.hpp:1182
struct FVector GetDOFFocusLoc(class AActor *TraceOwner, const struct FVector &StartTrace, const struct FVector &EndTrace)
void OnBecomeActive(class UGameCameraBase *OldCamera)
bool DoFullDamageToActor(class AActor *Victim)
bool DisplayMaterials(float X, float DY, class UMeshComponent *MeshComp, float *Y)
void IncrementCustomerCount(class AGameCrowdAgent *ArrivingAgent)
Definition: LA_Core_classes.hpp:1239
struct FColor DebugBehaviorColor
Definition: LA_GameFramework_classes.hpp:502
class AGameAIController * GameAIOwner
Definition: LA_GameFramework_classes.hpp:113
struct FVector ActualFocusPointWorldLoc
Definition: LA_GameFramework_classes.hpp:2197
unsigned long bUseRootMotion
Definition: LA_GameFramework_classes.hpp:1856
float CurrentBehaviorActivationTime
Definition: LA_GameFramework_classes.hpp:321
Definition: LA_Engine_classes.hpp:15115
Definition: LA_GameFramework_classes.hpp:2528
Definition: LA_Engine_classes.hpp:28280
Definition: LA_Engine_classes.hpp:4159
void DrawMobileTilt(class UMobilePlayerInput *MobileInput)
unsigned long bBlendBetweenAnims
Definition: LA_GameFramework_classes.hpp:544
Definition: LA_GameFramework_classes.hpp:1813
Definition: LA_Core_structs.hpp:236
struct FTextureUVs JoystickHatUVs
Definition: LA_GameFramework_classes.hpp:1180
class UPrimitiveComponent * SelectedComponent
Definition: LA_GameFramework_classes.hpp:1119
class AGameCrowdDestination * DebugSpawnDest
Definition: LA_GameFramework_classes.hpp:343
Definition: LA_Core_structs.hpp:696
float Tolerance
Definition: LA_GameFramework_classes.hpp:2098
unsigned long bIsActive
Definition: LA_GameFramework_classes.hpp:1901
unsigned long bAdjustDOF
Definition: LA_GameFramework_classes.hpp:2322
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2905
struct FVector InitialFinalDestination
Definition: LA_GameFramework_classes.hpp:2715
struct FVector LastActualCameraOrigin
Definition: LA_GameFramework_classes.hpp:2178
unsigned long bAllowCloudSpawning
Definition: LA_GameFramework_classes.hpp:749
float PenetrationBlockedPct
Definition: LA_GameFramework_classes.hpp:2175
void ModifyPostProcessSettings(struct FPostProcessSettings *PP)
void AddToMobileInput(class UMobilePlayerInput *MPI)
struct FString TargetZoneName
Definition: LA_GameFramework_classes.hpp:1968
unsigned long bSkipCameraCollision
Definition: LA_GameFramework_classes.hpp:2319
Definition: LA_GameFramework_classes.hpp:457
float DOF_MaxNearBlurAmount
Definition: LA_GameFramework_classes.hpp:2352
unsigned long bValidateWorstLoc
Definition: LA_GameFramework_classes.hpp:2318
Definition: LA_Engine_classes.hpp:13347
float CringeRadius
Definition: LA_GameFramework_classes.hpp:984
void Resumed(const struct FName &OldCommandName)
void AnalyzeSpawnPoints(int StartIndex, int NumToUpdate, struct FCrowdSpawnInfoItem *Item)
class UCameraShake * CamShake_Rear
Definition: LA_GameFramework_classes.hpp:2787
struct FVector GetAttemptedSpawnLocation(float Pct, const struct FVector &CurPos, float CurRadius, const struct FVector &DestPos, float DestRadius)
unsigned long bReachPreciseRotation
Definition: LA_GameFramework_classes.hpp:2422
float ExploRadialBlurFadeOutTime
Definition: LA_GameFramework_classes.hpp:2796
TArray< struct FBehaviorEntry > PanicBehaviors
Definition: LA_GameFramework_classes.hpp:329
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:3349
class UCylinderComponent * CylinderComponent
Definition: LA_GameFramework_classes.hpp:693
class UClass * NavigationHandleClass
Definition: LA_GameFramework_classes.hpp:852
float PENALTY_COEFF_ANGLETOVEL
Definition: LA_GameFramework_classes.hpp:283
void PlayAgentAnimationNow()
struct FMatrix LastTargetBaseTM
Definition: LA_GameFramework_classes.hpp:2275
float MaxAnimationDistance
Definition: LA_GameFramework_classes.hpp:430
Definition: LA_Core_structs.hpp:263
unsigned long bWantsGroupIdle
Definition: LA_GameFramework_classes.hpp:267
class URadialBlurComponent * ExploRadialBlur
Definition: LA_GameFramework_classes.hpp:2795
float NotVisibleLifeSpan
Definition: LA_GameFramework_classes.hpp:308
TArray< struct FName > AnimationList
Definition: LA_GameFramework_classes.hpp:538
TArray< int > AggregatesFound
Definition: LA_GameFramework_classes.hpp:2501
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1950
class UGameCrowdGroup * MyGroup
Definition: LA_GameFramework_classes.hpp:245
void AgentDestroyed(class AGameCrowdAgent *Agent)
int TurnEndAngle
Definition: LA_GameFramework_classes.hpp:2210
float Priority
Definition: LA_GameFramework_classes.hpp:774
float TraceDistance
Definition: LA_GameFramework_classes.hpp:1682
unsigned long bPassOnIsViralBehaviorFlag
Definition: LA_GameFramework_classes.hpp:493
unsigned long bWillBeVisible
Definition: LA_GameFramework_classes.hpp:755
void AdjustFocusPointInterpolation(const struct FRotator &Delta)
void ChangingDestination(class AGameCrowdDestination *NewDest)
struct FVector Rotation
Definition: LA_GameFramework_classes.hpp:1599
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:819
class UAnimNodeBlend * SpeedBlendNode
Definition: LA_GameFramework_classes.hpp:405
unsigned long bSimulateThisTick
Definition: LA_GameFramework_classes.hpp:264
float ExactReachTolerance
Definition: LA_GameFramework_classes.hpp:769
Definition: LA_GameFramework_classes.hpp:2392
void ToggleDebugCamera(bool bDrawDebugText)
class USoundCue * AmbientSoundCue
Definition: LA_GameFramework_classes.hpp:318
void PlayDeath(const struct FVector &KillMomentum)
void PopCommand(class UGameAICommand *ToBePoppedCommand)
bool AllowThisDestination(class AGameCrowdDestination *Destination)
class AActor * Attachee
Definition: LA_GameFramework_classes.hpp:1044
unsigned long bTurnAlignTargetWhenFinished
Definition: LA_GameFramework_classes.hpp:2203
Definition: LA_Engine_classes.hpp:3930
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2678
float DefaultFOV
Definition: LA_GameFramework_classes.hpp:2817
class AGamePawn * PawnOwner
Definition: LA_GameFramework_classes.hpp:2416
Definition: LA_Engine_classes.hpp:25968
Definition: LA_GameFramework_classes.hpp:2117
unsigned long bForceNavMeshPathing
Definition: LA_GameFramework_classes.hpp:1710
float ExplosionEmitterScale
Definition: LA_GameFramework_classes.hpp:988
unsigned long bHasNotifiedSpawner
Definition: LA_GameFramework_classes.hpp:270
class URadialBlurComponent * ExplosionRadialBlur
Definition: LA_GameFramework_classes.hpp:1032
void Explode(class UGameExplosion *NewExplosionTemplate, const struct FVector &Direction)
float SubGoalReachDist
Definition: LA_GameFramework_classes.hpp:2706
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1121
void HandleBehaviorEvent(TEnumAsByte< ECrowdBehaviorEvent > EventType, class AActor *InInstigator, bool bViralCause, bool bPropagateViralFlag)
Definition: LA_Core_structs.hpp:595
void OnBecomeInActive(class APawn *TargetPawn, class UGameThirdPersonCameraMode *NewMode)
class ADebugCameraController * DebugCameraControllerRef
Definition: LA_GameFramework_classes.hpp:219
struct FVector LastWorstLocation
Definition: LA_GameFramework_classes.hpp:2218
float PENALTY_COEFF_MAG
Definition: LA_GameFramework_classes.hpp:284
void EnableDebugCamera(bool bEnableDebugText)
Definition: LA_GameFramework_structs.hpp:468
float CameraLensEffectRadius
Definition: LA_GameFramework_classes.hpp:1009
float AnimVelRate
Definition: LA_GameFramework_classes.hpp:417
unsigned char UnknownData00[0x3]
Definition: LA_GameFramework_classes.hpp:274
struct FName UnselectKey
Definition: LA_GameFramework_classes.hpp:1110
int OldMobileGroup
Definition: LA_GameFramework_classes.hpp:2859
Definition: LA_GameFramework_classes.hpp:2900
struct FVector GetCameraWorstCaseLoc(class APawn *TargetPawn, const struct FTViewTarget &CurrentViewTarget)
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2748
bool AllowableDestinationFor(class AGameCrowdAgent *Agent)
class UObject * FinalTouchObject
Definition: LA_GameFramework_classes.hpp:2079
unsigned long bFinalApproach
Definition: LA_GameFramework_classes.hpp:2702
bool CanBeUsedBy(class AGameCrowdAgent *Agent, const struct FVector &CameraLoc)
float DesiredGroupRadiusSq
Definition: LA_GameFramework_classes.hpp:337
void OnActivate(class APlayerController *PC)
float LastProgressTime
Definition: LA_GameFramework_classes.hpp:286
class UCameraShake * CamShake
Definition: LA_GameFramework_classes.hpp:2784
void RemoveSpawnPoint(class AGameCrowdDestination *GCD)
float PlayerPositionPredictionTime
Definition: LA_GameFramework_classes.hpp:850
void UpdateCameraMode(class APawn *P)
int Focus_MaxTries
Definition: LA_GameFramework_classes.hpp:2194
void InternalTick(float DeltaTime)
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:187
class USoundCue * ExplosionSoundHurtSomeone
Definition: LA_GameFramework_classes.hpp:993
unsigned long bIsPlayingDeathAnimation
Definition: LA_GameFramework_classes.hpp:426
struct FVector DOFTrace(class AActor *TraceOwner, const struct FVector &StartTrace, const struct FVector &EndTrace)
float BlendTime
Definition: LA_GameFramework_classes.hpp:2309
unsigned long bAllowPerMaterialFX
Definition: LA_GameFramework_classes.hpp:966
class UDrawFrustumComponent * DrawFrustum
Definition: LA_GameFramework_classes.hpp:1117
Definition: LA_Engine_classes.hpp:4703
float OriginRotInterpSpeed
Definition: LA_GameFramework_classes.hpp:2332
float XL
Definition: LA_GameFramework_classes.hpp:3342
struct FVector BeaconOffset
Definition: LA_GameFramework_classes.hpp:315
int STATIC_GetObjClassVersion()
float SpeedBlendStart
Definition: LA_GameFramework_classes.hpp:415
float RunOffsetInterpSpeedOut
Definition: LA_GameFramework_classes.hpp:2343
float MobileTiltY
Definition: LA_GameFramework_classes.hpp:1192
unsigned long bAborted
Definition: LA_GameFramework_classes.hpp:117
void OnBecomeActive(class UGameCameraBase *OldCamera)
struct FRotator PreciseRotation
Definition: LA_GameFramework_classes.hpp:2429
struct FVector StrafeLeftAdjustment
Definition: LA_GameFramework_classes.hpp:2333
class UNavigationHandle * NavigationHandle
Definition: LA_GameFramework_classes.hpp:853
float OffsetAdjustmentInterpSpeed
Definition: LA_GameFramework_classes.hpp:2362
Definition: LA_Core_structs.hpp:227
float DOF_BlurKernelSize
Definition: LA_GameFramework_classes.hpp:2350
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:943
void OnAnimEnd(class UAnimNodeSequence *SeqNode, float PlayedTime, float ExcessTime)
float WalkableFloorZ
Definition: LA_GameFramework_classes.hpp:305
void DrawDebug(bool bPresistent, TArray< struct FCrowdSpawnerPlayerInfo > *PlayerInfo)
unsigned long bResetInterp
Definition: LA_GameFramework_classes.hpp:2270
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2035
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:778
struct FVector LastFocusPointLoc
Definition: LA_GameFramework_classes.hpp:2198
class AActor * LastTargetBase
Definition: LA_GameFramework_classes.hpp:2274
TArray< struct FTeamEvents > AllTeamEvents
Definition: LA_GameFramework_classes.hpp:2503
struct FInterpCurveVector2D AnimatedPosition
Definition: LA_GameFramework_classes.hpp:24
class UGameThirdPersonCameraMode * FindBestCameraMode(class APawn *P)
Definition: LA_Core_structs.hpp:253
unsigned long bPreferVisibleDestination
Definition: LA_GameFramework_classes.hpp:268
bool AllowStateTransitionTo(const struct FName &StateName)
void SetCurrentAnimationActionFor(class AGameCrowdAgentSkeletal *Agent)
struct FVector2D DOF_RadiusRange
Definition: LA_GameFramework_classes.hpp:2359
float DurationOfBehaviorEvent
Definition: LA_GameFramework_classes.hpp:714
Definition: LA_Engine_classes.hpp:4732
unsigned long bCanSpawnHereNow
Definition: LA_GameFramework_classes.hpp:756
Definition: LA_GameFramework_structs.hpp:284
float ConformTraceDist
Definition: LA_GameFramework_classes.hpp:275
Definition: LA_GameFramework_structs.hpp:326
struct FString GetDumpString()
Definition: LA_GameFramework_classes.hpp:1024
Definition: LA_GameFramework_classes.hpp:3275
Definition: LA_GameFramework_classes.hpp:710
void WaitForGroupMembers()
Definition: LA_GameFramework_classes.hpp:1571
void DelayedExplosionDamage()
float MaxYawRate
Definition: LA_GameFramework_classes.hpp:292
bool MoveUnreachable(const struct FVector &AttemptedDest, class AActor *AttemptedTarget)
void SetSoundMode(const struct FName &InSoundModeName)
class AActor * GetFocusActor()
TArray< class AGameCrowdDestination * > NextDestinations
Definition: LA_GameFramework_classes.hpp:760
float YL
Definition: LA_GameFramework_classes.hpp:3343
class UGameThirdPersonCamera * ThirdPersonCam
Definition: LA_GameFramework_classes.hpp:2307
unsigned long bOrientCameraShakeTowardsEpicenter
Definition: LA_GameFramework_classes.hpp:971
float DamageRadius
Definition: LA_GameFramework_classes.hpp:976
void DoForceFeedbackForScreenShake(class UCameraShake *ShakeData, float Scale)
Definition: LA_Engine_classes.hpp:14181
unsigned long bParticleSystemIsBeingOverriddenDontUsePhysMatVersion
Definition: LA_GameFramework_classes.hpp:967
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2765
unsigned long bWasFiring
Definition: LA_GameFramework_classes.hpp:2701
float MobileTiltSize
Definition: LA_GameFramework_classes.hpp:1193
void PostRenderFor(class APlayerController *PC, class UCanvas *Canvas, const struct FVector &CameraPosition, const struct FVector &CameraDir)
unsigned long bTickWhenNotVisible
Definition: LA_GameFramework_classes.hpp:424
float CamShakeInnerRadius
Definition: LA_GameFramework_classes.hpp:1005
unsigned long bCastShadows
Definition: LA_GameFramework_classes.hpp:1707
float LastCanDoSpecialMoveTime
Definition: LA_GameFramework_classes.hpp:2418
void UpdateCamera(class APawn *P, class AGamePlayerCamera *CameraActor, float DeltaTime, struct FTViewTarget *OutVT)
Definition: LA_Engine_classes.hpp:8926
void AddPrioritizedSpawnPoint(class AGameCrowdDestination *GCD, struct FCrowdSpawnInfoItem *Item)
void SpawnExplosionDecal()
Definition: LA_GameFramework_classes.hpp:2646
class UTexture2D * BeaconTexture
Definition: LA_GameFramework_classes.hpp:316
Definition: LA_GameFramework_classes.hpp:1082
TEnumAsByte< ETextDrawMethod > TextDrawMethod
Definition: LA_GameFramework_classes.hpp:3371
void ProcessViewRotation(float DeltaTime, class AActor *ViewTarget, struct FRotator *out_ViewRotation, struct FRotator *out_DeltaRot)
float MIN_PENALTY_THRESHOLD
Definition: LA_GameFramework_classes.hpp:285
class AGameCrowdAgent * MyArchetype
Definition: LA_GameFramework_classes.hpp:309
Definition: LA_GameFramework_structs.hpp:450
unsigned long bForceMobileHUD
Definition: LA_GameFramework_classes.hpp:1171
struct FLinearColor BeaconColor
Definition: LA_GameFramework_classes.hpp:317
void Touch(class AActor *Other, class UPrimitiveComponent *OtherComp, const struct FVector &HitLocation, const struct FVector &HitNormal)
void ProcessViewRotation(float DeltaTime, class AActor *ViewTarget, struct FRotator *out_ViewRotation, struct FRotator *out_DeltaRot)
Definition: LA_Engine_classes.hpp:11136
Definition: LA_GameFramework_classes.hpp:1920
struct FString GetBehaviorString()
TArray< struct FAvoidOtherSampleItem > AvoidOtherSampleList
Definition: LA_GameFramework_classes.hpp:281
unsigned long bIsVisible
Definition: LA_GameFramework_classes.hpp:754
bool STATIC_HoverToGoal(class AGameAIController *AI, class AActor *InGoal, float InGoalDistance, float InHoverHeight)
void ModifyPostProcessSettings(struct FPostProcessSettings *PP)
struct FVector PreciseDestRelOffset
Definition: LA_GameFramework_classes.hpp:2427
struct FVector FallbackDest
Definition: LA_GameFramework_classes.hpp:2712
int LastPostCamTurnYaw
Definition: LA_GameFramework_classes.hpp:2213
struct FLinearColor DisplayColor
Definition: LA_GameFramework_classes.hpp:3339
void ChangingDestination(class AGameCrowdDestination *NewDest)
class AActor * ActionTarget
Definition: LA_GameFramework_classes.hpp:494
void BeginTurn(int StartAngle, int EndAngle, float TimeSec, float DelaySec, bool bAlignTargetWhenFinished)
int CustomerCount
Definition: LA_GameFramework_classes.hpp:764
void InternalPaused(class UGameAICommand *NewCommand)
class UGameAICommand * GetAICommandInStack(class UClass *InClass)
class UGameAICommand * ChildCommand
Definition: LA_GameFramework_classes.hpp:111
class UGameExplosion * ExplosionTemplate
Definition: LA_GameFramework_classes.hpp:1039
unsigned long bClearingQueue
Definition: LA_GameFramework_classes.hpp:814
float OriginLocInterpSpeed
Definition: LA_GameFramework_classes.hpp:2330
Definition: LA_Engine_classes.hpp:10596
void SetRootMotion(bool bRootMotionEnabled)
unsigned long bFallbackMoveToMesh
Definition: LA_GameFramework_classes.hpp:2703
Definition: LA_GameFramework_classes.hpp:1897
void UpdatePostProcess(float DeltaTime, struct FTViewTarget *VT)
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2224
struct FInterpCurveFloat AnimatedScale
Definition: LA_GameFramework_classes.hpp:22
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1578
float DOF_RadiusFalloff
Definition: LA_GameFramework_classes.hpp:2358
unsigned long bNoFOVPostProcess
Definition: LA_GameFramework_classes.hpp:2324
class AActor * GetBehaviorInstigator()
bool UpdateSpawner(float DeltaTime, struct FCrowdSpawnInfoItem *Item)
Definition: LA_Core_structs.hpp:334
float LoopTime
Definition: LA_GameFramework_classes.hpp:1861
float ProximityLODDist
Definition: LA_GameFramework_classes.hpp:296
void OnAnimEnd(class UAnimNodeSequence *SeqNode, float PlayedTime, float ExcessTime)
void ResetInterpolation()
void NativePostRenderFor(class APlayerController *PC, class UCanvas *Canvas, const struct FVector &CameraPosition, const struct FVector &CameraDir)
Definition: LA_Basic.hpp:69
unsigned long bInterpLocation
Definition: LA_GameFramework_classes.hpp:2313
unsigned char UnknownData00[0x3]
Definition: LA_GameFramework_classes.hpp:2472
float DamageFalloffExponent
Definition: LA_GameFramework_classes.hpp:977
float StrafeOffsetScalingThreshold
Definition: LA_GameFramework_classes.hpp:2335
unsigned long bDirectLook
Definition: LA_GameFramework_classes.hpp:2311
float LastSpawnTime
Definition: LA_GameFramework_classes.hpp:775
void InitDebugInputSystem()
Definition: LA_GameFramework_classes.hpp:1880
class AGameCrowdDestination * PreviousDestination
Definition: LA_GameFramework_classes.hpp:250
Definition: LA_Engine_classes.hpp:27821
void DisplayDebug(class AHUD *HUD, float *out_YL, float *out_YPos)
float CurrentHoverHeight
Definition: LA_GameFramework_classes.hpp:2672
float RadialBlurFadeTime
Definition: LA_GameFramework_classes.hpp:1036
float ForceUpdateTime
Definition: LA_GameFramework_classes.hpp:333
Definition: LA_Engine_structs.hpp:3568
float DamageDelay
Definition: LA_GameFramework_classes.hpp:974
unsigned long bForceObstacleChecking
Definition: LA_GameFramework_classes.hpp:1709
float Roll
Definition: LA_GameFramework_classes.hpp:1943
bool HandlePathObstruction(class AActor *BlockedBy)
class UGameThirdPersonCameraMode * ThirdPersonCamDefault
Definition: LA_GameFramework_classes.hpp:2185
unsigned long bCheckonTouch
Definition: LA_GameFramework_classes.hpp:2076
void CrowdDebug(bool bEnabled)
class AActor * Find
Definition: LA_GameFramework_classes.hpp:2699
unsigned long bDoSeamlessPivotTransition
Definition: LA_GameFramework_classes.hpp:2207
unsigned long bExplodeMoreThanOnce
Definition: LA_GameFramework_classes.hpp:1028
class AActor * GetDestinationActor()
unsigned long bIsPanicked
Definition: LA_GameFramework_classes.hpp:266
float CamShakeFalloff
Definition: LA_GameFramework_classes.hpp:2790
unsigned long bPreferVisibleDestinationOnSpawn
Definition: LA_GameFramework_classes.hpp:269
void OnBecomeActive(class APawn *TargetPawn, class UGameThirdPersonCameraMode *PrevMode)
float Focus_FastAdjustKickInTime
Definition: LA_GameFramework_classes.hpp:2195
float AgentWarmupTime
Definition: LA_GameFramework_classes.hpp:1718
unsigned long bAIBroken
Definition: LA_GameFramework_classes.hpp:69
unsigned long bHasRunawayCommandList
Definition: LA_GameFramework_classes.hpp:63
struct FString ConsoleCommand(const struct FString &Command, bool bWriteToLog)
bool DoExplosionDamage(bool bCauseDamage, bool bCauseEffects)
void NotifyCrowdAgentRefresh()
void PrioritizeSpawnPoint(float MaxSpawnDist, TArray< struct FCrowdSpawnerPlayerInfo > *PlayerInfo)
unsigned long bUniformScale
Definition: LA_GameFramework_classes.hpp:257
unsigned long bPaused
Definition: LA_GameFramework_classes.hpp:272
Definition: LA_GameFramework_classes.hpp:2304
bool Warmup(int WarmupNum, struct FCrowdSpawnInfoItem *Item)
TArray< class AGameCrowdAgent * > Members
Definition: LA_GameFramework_classes.hpp:649
struct FViewOffsetData ViewOffset_ViewportAdjustments[0x6]
Definition: LA_GameFramework_classes.hpp:2348
class UGameCameraBase * ThirdPersonCam
Definition: LA_GameFramework_classes.hpp:2263
unsigned long bEnableCrowdLightEnvironment
Definition: LA_GameFramework_classes.hpp:1706
void InternalResumed(const struct FName &OldCommandName)
class AActor * GetBehaviorInstigator()
unsigned long bAvoidWhenPanicked
Definition: LA_GameFramework_classes.hpp:743
float AdjustFOVForViewport(float inHorizFOV, class APawn *CameraTargetPawn)
float ExploRadialBlurMaxBlur
Definition: LA_GameFramework_classes.hpp:998
bool IsBehindExplosion(class AActor *A)
float RadialBlurFadeTimeRemaining
Definition: LA_GameFramework_classes.hpp:1037
class AActor * PreciseDestBase
Definition: LA_GameFramework_classes.hpp:2426
unsigned long bAIDrawDebug
Definition: LA_GameFramework_classes.hpp:68
float Focus_StepHeightAdjustment
Definition: LA_GameFramework_classes.hpp:2193
class AActor * Goal
Definition: LA_GameFramework_classes.hpp:2668
struct FVector LastWorstLocationLocal
Definition: LA_GameFramework_classes.hpp:2217
void InitSpecialMove(class AGamePawn *inPawn, const struct FName &InHandle)
class USoundCue * ExplosionSound
Definition: LA_GameFramework_classes.hpp:992
Definition: LA_Engine_classes.hpp:24391
void Tick(float DeltaTime)
void OverlappedActorEvent(class AActor *A)
struct FVector LastKnownGoodPosition
Definition: LA_GameFramework_classes.hpp:298
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:3279
struct FVector HitLocation
Definition: LA_GameFramework_classes.hpp:1684
float PreciseRotationInterpolationTime
Definition: LA_GameFramework_classes.hpp:2428
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:77
void AILog_Internal(const struct FString &LogText, const struct FName &LogCategory, bool bForce)
float TurnDelay
Definition: LA_GameFramework_classes.hpp:2212
void OnBecomeActive(class UGameCameraBase *OldCamera)
int ConformTraceInterval
Definition: LA_GameFramework_classes.hpp:276
TEnumAsByte< EGameSessionType > SessionType
Definition: LA_GameFramework_classes.hpp:2471
float WorstLocPenetrationExtentScale
Definition: LA_GameFramework_classes.hpp:2172
struct FVector LastIdealCameraOrigin
Definition: LA_GameFramework_classes.hpp:2183
float BlendInTime
Definition: LA_GameFramework_classes.hpp:1854
class UCameraShake * CamShake
Definition: LA_GameFramework_classes.hpp:1001
class USeqAct_GameCrowdSpawner * Spawner
Definition: LA_GameFramework_classes.hpp:903
unsigned long bIsFrozenRendering
Definition: LA_GameFramework_classes.hpp:1113
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2400
void ReachedDestination(class AGameCrowdAgent *Agent)
Definition: LA_Engine_classes.hpp:19
void InitBehavior(class AGameCrowdAgent *Agent)
float SplitScreenShakeScale
Definition: LA_GameFramework_classes.hpp:2273
unsigned long bApplyControl
Definition: LA_GameFramework_classes.hpp:2123
unsigned long bShowMobileTilt
Definition: LA_GameFramework_classes.hpp:1172
float KnockDownStrength
Definition: LA_GameFramework_classes.hpp:983
int EndOfRenderingMovieFrame
Definition: LA_GameFramework_classes.hpp:1662
class UTexture2D * JoystickBackground
Definition: LA_GameFramework_classes.hpp:1177
unsigned long bAutoControllerVibration
Definition: LA_GameFramework_classes.hpp:2781
float FractureMeshRadius
Definition: LA_GameFramework_classes.hpp:999
struct FString MovieName
Definition: LA_GameFramework_classes.hpp:1660
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2510
TEnumAsByte< ECrowdBehaviorEvent > EventType
Definition: LA_GameFramework_classes.hpp:715
class UGameCameraBase * FixedCam
Definition: LA_GameFramework_classes.hpp:2265
class APlayerController * Login(const struct FString &Portal, const struct FString &Options, const struct FUniqueNetId &UniqueId, struct FString *ErrorMessage)
Definition: LA_Engine_classes.hpp:13732
Definition: LA_GameFramework_classes.hpp:2260
Definition: LA_GameFramework_classes.hpp:1657
float OriginOffsetInterpSpeed
Definition: LA_GameFramework_classes.hpp:2180
unsigned long bUseOverlapCheck
Definition: LA_GameFramework_classes.hpp:970
struct FVector LastMovePoint
Definition: LA_GameFramework_classes.hpp:2709
void DisplayDebug(class AHUD *HUD, float *out_YL, float *out_YPos)
bool AllowBehaviorAt(class AGameCrowdDestination *Destination)
void PushCommand(class UGameAICommand *NewCommand)
void SpecialMoveEnded(const struct FName &PrevMove, const struct FName &NextMove)
void RemoveMember(class AGameCrowdAgent *Agent)
Definition: LA_Engine_classes.hpp:13866
class AFogVolumeSphericalDensityInfo * FogVolumeArchetype
Definition: LA_GameFramework_classes.hpp:2799
Definition: LA_GameFramework_classes.hpp:807
class UGameThirdPersonCameraMode * CreateCameraMode(class UClass *ModeClass)
class UGameAICommand * FindCommandOfClass(class UClass *SearchClass)
struct FTextureUVs JoystickBackgroundUVs
Definition: LA_GameFramework_classes.hpp:1178
unsigned long bIgnoreNotifies
Definition: LA_GameFramework_classes.hpp:118
class AActor * CustomActionTarget
Definition: LA_GameFramework_classes.hpp:545
unsigned long bSkipDefaultPhysMatParticleSystem
Definition: LA_GameFramework_classes.hpp:968
float Radius
Definition: LA_GameFramework_classes.hpp:2669
void SetViewOffset(struct FViewOffsetData *NewViewOffset)
Definition: LA_Engine_classes.hpp:9788
void UpdateShadowSettings(bool bInWantShadow)
class UFont * DisplayFont
Definition: LA_GameFramework_classes.hpp:3367
struct FPawnEvents AllPawnEvents
Definition: LA_GameFramework_classes.hpp:2507
unsigned long bDirectionalExplosion
Definition: LA_GameFramework_classes.hpp:959
class UClass * ActorClassToIgnoreForKnockdownsAndCringes
Definition: LA_GameFramework_classes.hpp:980
TArray< class AGameCrowdDestination * > GlobalPotentialSpawnPoints
Definition: LA_GameFramework_classes.hpp:848
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1603
unsigned long bFlushAILogEachLine
Definition: LA_GameFramework_classes.hpp:66
float STATIC_BoxDistanceToPoint(const struct FVector &Start, const struct FBox &BBox)
float RotateToTargetSpeed
Definition: LA_GameFramework_classes.hpp:291
float LastUpdateTime
Definition: LA_GameFramework_classes.hpp:307
class AGameCrowdAgent * CreateNewAgent(class AGameCrowdDestination *SpawnLoc, class AGameCrowdAgent *AgentTemplate, class UGameCrowdGroup *NewGroup, struct FCrowdSpawnInfoItem *Item)
float AverageReactionTime
Definition: LA_GameFramework_classes.hpp:816
bool AllowThisDestination(class AGameCrowdDestination *Destination)
void InitBehavior(class AGameCrowdAgent *Agent)
bool ShouldIgnoreNotifies()
struct FVector MeshMinScale3D
Definition: LA_GameFramework_classes.hpp:293
struct FVector SearchExtent
Definition: LA_GameFramework_classes.hpp:301
class USeqAct_PlayAgentAnimation * AnimSequence
Definition: LA_GameFramework_classes.hpp:548
Definition: LA_GameFramework_classes.hpp:1850
float ViewOffsetInterp
Definition: LA_GameFramework_classes.hpp:2361
struct FRotator LastActualCameraOriginRot
Definition: LA_GameFramework_classes.hpp:2179
Definition: LA_GameFramework_classes.hpp:1740
void SpecialMoveStarted(bool bForced, const struct FName &PrevMove)
unsigned long bMapBasedLogName
Definition: LA_GameFramework_classes.hpp:67
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:345
struct FVector FinalTouchNormal
Definition: LA_GameFramework_classes.hpp:2078
struct FVector GetActualFocusLocation()
Definition: LA_GameFramework_classes.hpp:2168
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:720
unsigned long bPlayRecoil
Definition: LA_GameFramework_classes.hpp:2121
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2532
void SpecialPawnEffectsFor(class AGamePawn *VictimPawn, float VictimDist)
float InteractionDelay
Definition: LA_GameFramework_classes.hpp:771
float WorstLocAimingZOffset
Definition: LA_GameFramework_classes.hpp:2396
int Health
Definition: LA_GameFramework_classes.hpp:252
float CurrentPathLaneValue
Definition: LA_GameFramework_classes.hpp:289
Definition: LA_GameFramework_classes.hpp:2607
Definition: LA_Engine_classes.hpp:23193
bool STATIC_BiasAgainstPolysWithinDistanceOfLocations(class UNavigationHandle *NavHandle, const struct FVector &InLocation, const struct FRotator &InRotation, float InDistanceToCheck, TArray< struct FVector > InLocationsToCheck)
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2277
struct FVector PreciseDestination
Definition: LA_GameFramework_classes.hpp:2425
TEnumAsByte< EConformType > ConformType
Definition: LA_GameFramework_classes.hpp:273
Definition: LA_GameFramework_classes.hpp:59
struct FRecoilDef Recoil
Definition: LA_GameFramework_classes.hpp:2124
Definition: LA_Core_structs.hpp:296
class UTexture2D * SliderImages[0x4]
Definition: LA_GameFramework_classes.hpp:1189
int NumAgentsToTickPerFrame
Definition: LA_GameFramework_classes.hpp:1719
void SetColorScale(const struct FVector &NewColorScale)
int CreateSpawner(class USeqAct_GameCrowdPopulationManagerToggle *inAction)
void SetLighting(bool bEnableLightEnvironment, const struct FLightingChannelContainer &AgentLightingChannel, bool bCastShadows)
class AActor * GetDestinationActor()
unsigned long bFaceActionTargetFirst
Definition: LA_GameFramework_classes.hpp:1857
class UClass * FixedCameraClass
Definition: LA_GameFramework_classes.hpp:2266
class UPointLightComponent * ExploLight
Definition: LA_GameFramework_classes.hpp:994
float NotVisibleDisableTickTime
Definition: LA_GameFramework_classes.hpp:429
unsigned long bIndividualSpawner
Definition: LA_GameFramework_classes.hpp:1711
float SubGoalReachDist
Definition: LA_GameFramework_classes.hpp:2673
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1970
void DrawMobileZone_Button(class UMobileInputZone *Zone)
void GetDebugOverheadText(class APlayerController *PC, TArray< struct FString > *OutText)
void ActivateBehavior(class UGameCrowdAgentBehavior *NewBehaviorArchetype, class AActor *LookAtActor)
struct FString DebugCameraControllerClassName
Definition: LA_GameFramework_classes.hpp:221
unsigned long bStoppingBehavior
Definition: LA_GameFramework_classes.hpp:623
float MaxAnimationDistanceSq
Definition: LA_GameFramework_classes.hpp:431
void RefreshKismetLinks()
void ClearFocusPoint(bool bLeaveCameraRotation)
float TurnTotalTime
Definition: LA_GameFramework_classes.hpp:2211
void DisplayDebug(class AHUD *HUD, float *out_YL, float *out_YPos)
unsigned long bLookAtPlayer
Definition: LA_GameFramework_classes.hpp:542
class UGameCrowd_ListOfAgents * CrowdAgentList
Definition: LA_GameFramework_classes.hpp:1704
unsigned long bLooping
Definition: LA_GameFramework_classes.hpp:543
Definition: LA_Core_structs.hpp:359
unsigned long bLineSpawner
Definition: LA_GameFramework_classes.hpp:751
unsigned long bShowMobileHud
Definition: LA_GameFramework_classes.hpp:1170
TArray< class AGameCrowdAgent * > LastSpawnedList
Definition: LA_GameFramework_classes.hpp:1720
unsigned long bAllowPitching
Definition: LA_GameFramework_classes.hpp:261
float DeadBodyDuration
Definition: LA_GameFramework_classes.hpp:253
bool GetAggregateMappingIDs(int EventID, int *AggregateID, int *TargetAggregateID)
TArray< class UObject * > SupportedArchetypes
Definition: LA_GameFramework_classes.hpp:766
class USoundCue * ExplosionSound
Definition: LA_GameFramework_classes.hpp:2783
void DrawMobileDebugString(float XPos, float YPos, const struct FString &Str)
class AGameCrowdAgent * SpawnAgentByIdx(int SpawnerIdx, class AGameCrowdDestination *SpawnLoc)
bool IsEnemyBasedOnInterpActor(class APawn *InEnemy)
unsigned long bDebugTouches
Definition: LA_GameFramework_classes.hpp:1173
Definition: LA_GameFramework_classes.hpp:1700
unsigned long bFullDamageToAttachee
Definition: LA_GameFramework_classes.hpp:962
class UCameraShake * CamShake_Right
Definition: LA_GameFramework_classes.hpp:2786
struct FString DemoActionString
Definition: LA_GameFramework_classes.hpp:75
class UClass * HUDClass
Definition: LA_GameFramework_classes.hpp:1114
void DoExplosionCameraEffects()
bool PickBehaviorFrom(TArray< struct FBehaviorEntry > BehaviorList, const struct FVector &BestCameraLoc)
int MaxMovePointFails
Definition: LA_GameFramework_classes.hpp:2711
float Pitch
Definition: LA_GameFramework_classes.hpp:1944
bool ReachedByAgent(class AGameCrowdAgent *Agent, const struct FVector &TestPosition, bool bTestExactly)
void TakeDamage(int DamageAmount, class AController *EventInstigator, const struct FVector &HitLocation, const struct FVector &Momentum, class UClass *DamageType, const struct FTraceHitInfo &HitInfo, class AActor *DamageCauser)
float LeftoverPitchAdjustment
Definition: LA_GameFramework_classes.hpp:2191
struct FVector RunFwdAdjustment
Definition: LA_GameFramework_classes.hpp:2339
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2631
float StrafeOffsetInterpSpeedOut
Definition: LA_GameFramework_classes.hpp:2337
Definition: LA_GameFramework_classes.hpp:737
struct FVector TargetRelativeCameraOriginOffset
Definition: LA_GameFramework_classes.hpp:2346
struct FVector PreferredVelocity
Definition: LA_GameFramework_classes.hpp:246
struct FArray_Mirror TeamStates
Definition: LA_GameFramework_classes.hpp:2469
void ClientPlayMovie(const struct FString &MovieName, int InStartOfRenderingMovieFrame, int InEndOfRenderingMovieFrame, bool bRestrictPausing, bool bPlayOnceFromStream, bool bOnlyBackButtonSkipsMovie)
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1088
float SplitScreenNumReduction
Definition: LA_GameFramework_classes.hpp:849
unsigned long bIsWarmupPaused
Definition: LA_GameFramework_classes.hpp:183
unsigned long bDoPredictiveAvoidance
Definition: LA_GameFramework_classes.hpp:2317
Definition: LA_GameFramework_classes.hpp:646
unsigned long bSkipBehaviorIfPanicked
Definition: LA_GameFramework_classes.hpp:744
unsigned long bDebugZones
Definition: LA_GameFramework_classes.hpp:1174
float Focus_BackOffStrength
Definition: LA_GameFramework_classes.hpp:2192
float RunOffsetInterpSpeedIn
Definition: LA_GameFramework_classes.hpp:2342
bool AllowBehaviorAt(class AGameCrowdDestination *Destination)
Definition: LA_GameFramework_classes.hpp:2761
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2717
float HeadVisibilityOffset
Definition: LA_GameFramework_classes.hpp:851
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:165
void SetCurrentBehavior(class UGameCrowdAgentBehavior *BehaviorArchetype)
Definition: LA_GameFramework_structs.hpp:348
Definition: LA_GameFramework_classes.hpp:2095
class UClass * NavigationHandleClass
Definition: LA_GameFramework_classes.hpp:302
Definition: LA_GameFramework_classes.hpp:598
unsigned long bWarnCrowdMembers
Definition: LA_GameFramework_classes.hpp:181
float DistanceToCheck
Definition: LA_GameFramework_classes.hpp:1600
class UClass * CameraLensEffect
Definition: LA_GameFramework_classes.hpp:1008
void UpdateIntermediatePoint(class AActor *DestinationActor)
Definition: LA_Basic.hpp:249
bool CanDoSpecialMove(bool bForceCheck)
float AgentAwareRadius
Definition: LA_GameFramework_classes.hpp:184
TArray< struct FName > AILogFilter
Definition: LA_GameFramework_classes.hpp:74
class UTexture2D * TrackballBackground
Definition: LA_GameFramework_classes.hpp:1185
float DurationBeforeBecomesViral
Definition: LA_GameFramework_classes.hpp:497
void UpdateExplosionTemplateWithPerMaterialFX(class UPhysicalMaterial *PhysMaterial)
class AGameCrowdDestination * CurrentDestination
Definition: LA_GameFramework_classes.hpp:248
TArray< struct FNearbyDynamicItem > NearbyDynamics
Definition: LA_GameFramework_classes.hpp:256
TArray< struct FPropertyInfo > Properties
Definition: LA_GameFramework_classes.hpp:1835
Definition: LA_GameFramework_classes.hpp:19
float GroundOffset
Definition: LA_GameFramework_classes.hpp:299
Definition: LA_GameFramework_classes.hpp:2140
float PenetrationExtentScale
Definition: LA_GameFramework_classes.hpp:2176
struct FTextureUVs TrackballBackgroundUVs
Definition: LA_GameFramework_classes.hpp:1186
unsigned long bHasRestrictions
Definition: LA_GameFramework_classes.hpp:747
Definition: LA_Engine_classes.hpp:33172
Definition: LA_Engine_classes.hpp:11256
struct FInterpCurveLinearColor AnimatedColor
Definition: LA_GameFramework_classes.hpp:23
float FracturePartVel
Definition: LA_GameFramework_classes.hpp:1000
float MaxSeePlayerDistSq
Definition: LA_GameFramework_classes.hpp:324
struct FVector MoveVectDest
Definition: LA_GameFramework_classes.hpp:2675
class UMobilePlayerInput * MPI
Definition: LA_GameFramework_classes.hpp:2860
struct FLightingChannelContainer AgentLightingChannel
Definition: LA_GameFramework_classes.hpp:1714
class AGameCrowdAgent * SpawnAgent(class AGameCrowdDestination *SpawnLoc, struct FCrowdSpawnInfoItem *Item)
float Radius
Definition: LA_GameFramework_classes.hpp:2700
TArray< struct FBehaviorEntry > AlertBehaviors
Definition: LA_GameFramework_classes.hpp:328
class UCameraShake * ChooseCameraShake(const struct FVector &Epicenter, class APlayerController *PC)
unsigned long bDebugCrowdAwareness
Definition: LA_GameFramework_classes.hpp:182
struct FString GetBehaviorString()
class URB_RadialImpulseComponent * RadialImpulseComponent
Definition: LA_GameFramework_classes.hpp:1040
Definition: LA_Engine_classes.hpp:11401
struct FVector LastViewOffset
Definition: LA_GameFramework_classes.hpp:2181
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1837
void UpdateCameraLensEffects(struct FTViewTarget *OutVT)
void CacheLastTargetBaseInfo(class AActor *TargetBase)
void InternalPrePushed(class AGameAIController *AI)
void AllCommands(class UClass *BaseClass, class UGameAICommand **Cmd)
Definition: LA_GameFramework_classes.hpp:572
Definition: LA_GameFramework_classes.hpp:3296
float Yaw
Definition: LA_GameFramework_classes.hpp:2031
int DestroyAllCount
Definition: LA_GameFramework_classes.hpp:905
void ReplicatedEvent(const struct FName &VarName)
struct FArray_Mirror PlayerStates
Definition: LA_GameFramework_classes.hpp:2470
unsigned long bInterpolateCamChanges
Definition: LA_GameFramework_classes.hpp:2269
Definition: LA_Engine_classes.hpp:24140
float GetDestinationOffset()
float RunOffsetScalingThreshold
Definition: LA_GameFramework_classes.hpp:2341
void GetCurrentMovie(struct FString *MovieName)
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1744
float DurationOfViralBehaviorPropagation
Definition: LA_GameFramework_classes.hpp:499
class UObject * HitObject
Definition: LA_GameFramework_classes.hpp:1683
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:651
unsigned long bDebugZonePresses
Definition: LA_GameFramework_classes.hpp:1175
Definition: LA_GameFramework_classes.hpp:1105
float DesiredHoverHeight
Definition: LA_GameFramework_classes.hpp:2704
TArray< struct FCrowdSpawnerPlayerInfo > PlayerInfo
Definition: LA_GameFramework_classes.hpp:855
bool AnalyzeSpawnPoint(float MaxSpawnDistSq, bool bForceNavMeshPathing, class UNavigationHandle *NavHandle, TArray< struct FCrowdSpawnerPlayerInfo > *PlayerInfo)
Definition: LA_GameFramework_classes.hpp:668
class AActor * HitActorFromPhysMaterialTrace
Definition: LA_GameFramework_classes.hpp:1042
Definition: LA_Engine_classes.hpp:1283
Definition: LA_GameFramework_structs.hpp:646
unsigned long bIdleBehavior
Definition: LA_GameFramework_classes.hpp:490
float DeltaYaw
Definition: LA_GameFramework_classes.hpp:1948
float LightFadeTimeRemaining
Definition: LA_GameFramework_classes.hpp:1034
class AActor * Initiator
Definition: LA_GameFramework_classes.hpp:718
class AGameCrowdDestinationQueuePoint * NextQueuePosition
Definition: LA_GameFramework_classes.hpp:810
float LastPlayerInfoUpdateTime
Definition: LA_GameFramework_classes.hpp:856
float MaxSpawnDist
Definition: LA_GameFramework_classes.hpp:1715
float DirectionalExplosionAngleDeg
Definition: LA_GameFramework_classes.hpp:973
Definition: LA_Engine_classes.hpp:11525
struct FVector DOFTraceExtent
Definition: LA_GameFramework_classes.hpp:2357
float TimeToStopPropagatingViralBehavior
Definition: LA_GameFramework_classes.hpp:500
Definition: LA_Engine_classes.hpp:2203
float DOF_FalloffExponent
Definition: LA_GameFramework_classes.hpp:2349
void ResetInterpolation()
Definition: LA_GameFramework_classes.hpp:1166
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1049
Definition: LA_GameFramework_structs.hpp:490
float WarmupPopulationPct
Definition: LA_GameFramework_classes.hpp:1703
unsigned long bDOFUpdated
Definition: LA_GameFramework_classes.hpp:2323
float TraceDistance
Definition: LA_GameFramework_classes.hpp:2102
void InitBehavior(class AGameCrowdAgent *Agent)
class UTexture2D * ButtonImages[0x2]
Definition: LA_GameFramework_classes.hpp:1181
bool ValidateSpawnAt(class AGameCrowdDestination *Candidate, struct FCrowdSpawnInfoItem *Item)
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1924
struct FPointer VfTable_IInterface_RVO
Definition: LA_GameFramework_classes.hpp:244
unsigned long bUseForcedCamFOV
Definition: LA_GameFramework_classes.hpp:2268
unsigned long bDrawDebug
Definition: LA_GameFramework_classes.hpp:2204
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:46
float LastCamFOV
Definition: LA_GameFramework_classes.hpp:2182
class AGameCrowdInteractionPoint * PreviousQueuePosition
Definition: LA_GameFramework_classes.hpp:811
Definition: LA_GameFramework_classes.hpp:1619
TArray< struct FName > IdleAnimNames
Definition: LA_GameFramework_classes.hpp:413
void STATIC_KeepPlayingLoadingMovie()
void SetDesiredRotation(const struct FRotator &TargetDesiredRotation, bool InLockDesiredRotation, bool InUnlockWhenReached, float InterpolationTime)
class AGamePlayerCamera * PlayerCamera
Definition: LA_GameFramework_classes.hpp:2143
void Tick(float DeltaTime)
void FlushAgents(const struct FCrowdSpawnInfoItem &Item)
unsigned long bMustReachExactly
Definition: LA_GameFramework_classes.hpp:746
struct FVector LastStrafeOffset
Definition: LA_GameFramework_classes.hpp:2338
TArray< struct FBehaviorEntry > EncounterAgentBehaviors
Definition: LA_GameFramework_classes.hpp:322
unsigned char UnknownData00[0x2]
Definition: LA_GameFramework_classes.hpp:487
Definition: LA_Engine_structs.hpp:8063
Definition: LA_GameFramework_classes.hpp:900
unsigned long bShowSelectedInfo
Definition: LA_GameFramework_classes.hpp:1111
float Frequency
Definition: LA_GameFramework_classes.hpp:763
unsigned long bReplaceActiveSameClassInstance
Definition: LA_GameFramework_classes.hpp:116
void DecrementCustomerCount(class AGameCrowdAgent *DepartingAgent)
class UClass * MyDamageType
Definition: LA_GameFramework_classes.hpp:981
struct FVector2D Aim
Definition: LA_GameFramework_classes.hpp:2125
float LastYawAdjustment
Definition: LA_GameFramework_classes.hpp:2190
unsigned long bCheckForObstacles
Definition: LA_GameFramework_classes.hpp:258
void FellOutOfWorld(class UClass *dmgType)
unsigned long bHasNavigationMesh
Definition: LA_GameFramework_classes.hpp:759
struct FVector Location
Definition: LA_GameFramework_classes.hpp:1598
float GoalDistance
Definition: LA_GameFramework_classes.hpp:2707
float RadialBlurMaxBlurAmount
Definition: LA_GameFramework_classes.hpp:1038
TArray< class AGameCrowdDestination * > PotentialSpawnPoints
Definition: LA_GameFramework_classes.hpp:671
int ConformTraceFrameCount
Definition: LA_GameFramework_classes.hpp:255
Definition: LA_GameFramework_classes.hpp:161
class UGameCrowdAgentBehavior * CurrentBehavior
Definition: LA_GameFramework_classes.hpp:320
bool CanChainMove(const struct FName &NextMove)
class AActor * HitActor
Definition: LA_GameFramework_classes.hpp:989
unsigned long bResetCameraInterpolation
Definition: LA_GameFramework_classes.hpp:2144
struct FVector SpawnOffset
Definition: LA_GameFramework_classes.hpp:340
struct FVector WorstLocOffset
Definition: LA_GameFramework_classes.hpp:2345
float FOVAngle
Definition: LA_GameFramework_classes.hpp:2308
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2650
struct FRotator LastPreModifierCameraRot
Definition: LA_GameFramework_classes.hpp:2220
Definition: LA_GameFramework_classes.hpp:689
struct FVector RotationVector
Definition: LA_GameFramework_classes.hpp:2033
Definition: LA_GameFramework_classes.hpp:2835
void PrimarySelect(const struct FVector &HitLoc, const struct FVector &HitNormal, const struct FTraceHitInfo &HitInfo)
Definition: LA_Engine_classes.hpp:13800
unsigned long bAdjacentToVisibleNode
Definition: LA_GameFramework_classes.hpp:758
struct FString GetDestString()
TEnumAsByte< ECrowdBehaviorEvent > MyEventType
Definition: LA_GameFramework_classes.hpp:485
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2146
struct FColor DebugAgentColor
Definition: LA_GameFramework_classes.hpp:342
Definition: LA_Basic.hpp:157
float FollowingInterpSpeed_Yaw
Definition: LA_GameFramework_classes.hpp:2327
class AActor * Find
Definition: LA_GameFramework_classes.hpp:2667
class AGameCrowdInfoVolume * ActiveCrowdInfoVolume
Definition: LA_GameFramework_classes.hpp:847
TArray< class UObject * > Targets
Definition: LA_GameFramework_classes.hpp:2080
void AddKismetRenderEvent(class USeqEvent_HudRender *NewEvent)
int RoundNumber
Definition: LA_GameFramework_classes.hpp:2475
float VL
Definition: LA_GameFramework_classes.hpp:3347
class UGameCameraBase * FindBestCameraType(class AActor *CameraTarget)
float LastFocusChangeTime
Definition: LA_GameFramework_classes.hpp:2196
void PostRender(class UCanvas *Canvas)
Definition: LA_Engine_classes.hpp:26439
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1153
float LightFadeTime
Definition: LA_GameFramework_classes.hpp:1033
class AActor * Path
Definition: LA_GameFramework_classes.hpp:2666
void Tick(float DeltaTime)
TArray< class UClass * > SupportedAgentClasses
Definition: LA_GameFramework_classes.hpp:765
Definition: LA_Engine_classes.hpp:23106
void InitDebugInputSystem()
float LastFallbackActiveTime
Definition: LA_GameFramework_classes.hpp:287
class APlayerController * OriginalControllerRef
Definition: LA_GameFramework_classes.hpp:1115
class UAnimTree * AgentTree
Definition: LA_GameFramework_classes.hpp:410
unsigned long bIsViralBehavior
Definition: LA_GameFramework_classes.hpp:492
struct FVector FinalTouchLocation
Definition: LA_GameFramework_classes.hpp:2077
int LoopIndex
Definition: LA_GameFramework_classes.hpp:1860
class UGameCameraBase * CreateCamera(class UClass *CameraClass)
void ChangeDebugColor(const struct FColor &InC)
float DirectLookInterpSpeed
Definition: LA_GameFramework_classes.hpp:2215
void SpecialCringeEffectsFor(class AActor *Victim, float VictimDist)
float ExploRadialBlurMaxBlur
Definition: LA_GameFramework_classes.hpp:2797
void SetLighting(bool bEnableLightEnvironment, const struct FLightingChannelContainer &AgentLightingChannel, bool bCastShadows)
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1623
bool ShouldDebugDestinations()
float Yaw
Definition: LA_GameFramework_classes.hpp:1945
unsigned long bDebugSpawns
Definition: LA_GameFramework_classes.hpp:857
float DOF_MaxFarBlurAmount
Definition: LA_GameFramework_classes.hpp:2353
unsigned long bDrawDebug
Definition: LA_GameFramework_classes.hpp:1030
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1817
bool STATIC_InitCommandUserActor(class AGameAIController *AI, class AActor *UserActor)
void FillCrowdSpawnInfoItem(class AGameCrowdPopulationManager *PopMgr, struct FCrowdSpawnInfoItem *out_Item)
class AGameCrowdAgent * MyAgent
Definition: LA_GameFramework_classes.hpp:501
Definition: LA_Basic.hpp:268
class UGameAICommand * CommandList
Definition: LA_GameFramework_classes.hpp:62
struct FVector HitNormal
Definition: LA_GameFramework_classes.hpp:991
class URadialBlurComponent * ExploRadialBlur
Definition: LA_GameFramework_classes.hpp:996
int TurnStartAngle
Definition: LA_GameFramework_classes.hpp:2209
TArray< class AGameCrowdDestination * > PotentialSpawnPoints
Definition: LA_GameFramework_classes.hpp:1717
float TimeUntilStopBehavior
Definition: LA_GameFramework_classes.hpp:489
void UpdateCamera(class APawn *P, class AGamePlayerCamera *CameraActor, float DeltaTime, struct FTViewTarget *OutVT)
unsigned long bPerformRadialBlurRelevanceCheck
Definition: LA_GameFramework_classes.hpp:964
unsigned long bInterpViewOffsetOnlyForCamTransition
Definition: LA_GameFramework_classes.hpp:2325
int STATIC_GetObjClassVersion()
void GetSpawnPosition(class USeqAct_GameCrowdSpawner *Spawner, struct FVector *SpawnPos, struct FRotator *SpawnRot)
Definition: LA_Core_structs.hpp:392
bool ShouldUpdateBreadCrumbs()
struct FString DisplayText
Definition: LA_GameFramework_classes.hpp:3370
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2819
struct FVector StrafeRightAdjustment
Definition: LA_GameFramework_classes.hpp:2334
Definition: LA_GameFramework_classes.hpp:2778
void SpawnExplosionFogVolume()
int STATIC_GetObjClassVersion()
struct FVector MeshMaxScale3D
Definition: LA_GameFramework_classes.hpp:294
float MaxSpeed
Definition: LA_GameFramework_classes.hpp:312
struct FVector RunBackAdjustment
Definition: LA_GameFramework_classes.hpp:2340
unsigned long bAnimateThisTick
Definition: LA_GameFramework_classes.hpp:428
Definition: LA_GameFramework_classes.hpp:401
Definition: LA_Engine_structs.hpp:3592
void ClientStopMovie(float DelayInSeconds, bool bAllowMovieToFinish, bool bForceStopNonSkippable, bool bForceStopLoadingMovie)
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1864
class AGameCrowdDestination * PickSpawnPoint(struct FCrowdSpawnInfoItem *Item)
class UPhysicalMaterial * GetPhysicalMaterial()
float ExploLightFadeOutTime
Definition: LA_GameFramework_classes.hpp:995
Definition: LA_GameFramework_classes.hpp:41
TArray< struct FGameCrowdAttachmentList > Attachments
Definition: LA_GameFramework_classes.hpp:420
float UL
Definition: LA_GameFramework_classes.hpp:3346
float InterpZTranslation
Definition: LA_GameFramework_classes.hpp:251
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2611
void ClearLatentAnimation()
void AddCustomer(class AGameCrowdAgent *NewCustomer, class AGameCrowdInteractionPoint *PreviousPosition)
class UAnimNodeSequence * RunSeqNode
Definition: LA_GameFramework_classes.hpp:409
Definition: LA_Engine_classes.hpp:33039
unsigned long bForcePrecisePosition
Definition: LA_GameFramework_classes.hpp:2424
Definition: LA_GameFramework_classes.hpp:216
Definition: LA_Engine_classes.hpp:6076
void OnPlayAgentAnimation(class USeqAct_PlayAgentAnimation *Action)
float LastGroundZ
Definition: LA_GameFramework_classes.hpp:278
unsigned long bPendingAdvance
Definition: LA_GameFramework_classes.hpp:815
int NumberOfClientsToWaitFor
Definition: LA_GameFramework_classes.hpp:3299
unsigned long bAutoControllerVibration
Definition: LA_GameFramework_classes.hpp:972
struct FVector PerAxisOriginLocInterpSpeed
Definition: LA_GameFramework_classes.hpp:2331
void Render(class UCanvas *TargetCanvas, class AHUD *TargetHud)
struct FVector RelativeToWorldOffset(const struct FRotator &InRotation, const struct FVector &RelativeSpaceOffset)
unsigned long bIsMatchStarted
Definition: LA_GameFramework_classes.hpp:2473
class AActor * ActionTarget
Definition: LA_GameFramework_classes.hpp:1862
class UMaterialInstanceConstant * MeshColor
Definition: LA_GameFramework_classes.hpp:461
class AGameCrowdPopulationManager * MyPopMgr
Definition: LA_GameFramework_classes.hpp:776
Definition: LA_Engine_classes.hpp:5601
Definition: LA_GameFramework_classes.hpp:1832
Definition: LA_GameFramework_structs.hpp:316
void UpdateViewTarget(float DeltaTime, struct FTViewTarget *OutVT)
float LastDOFDistance
Definition: LA_GameFramework_classes.hpp:2355
float DOFDistanceInterpSpeed
Definition: LA_GameFramework_classes.hpp:2356
void AgentDestroyed(class AGameCrowdAgent *Agent)
Definition: LA_GameFramework_structs.hpp:422
unsigned long bSpawningActive
Definition: LA_GameFramework_classes.hpp:904
unsigned long bReachPreciseDestination
Definition: LA_GameFramework_classes.hpp:2420
Definition: LA_Engine_classes.hpp:23338
TScriptInterface< class UGameCrowdSpawnerInterface > MySpawner
Definition: LA_GameFramework_classes.hpp:339
unsigned long bIgnoreStepAside
Definition: LA_GameFramework_classes.hpp:119
class UClass * ThirdPersonCamDefaultClass
Definition: LA_GameFramework_classes.hpp:2186
float TraceDistance
Definition: LA_GameFramework_classes.hpp:2075
struct FString GetBehaviorString()
struct FName SecondaryKey
Definition: LA_GameFramework_classes.hpp:1109
float ExploLightFadeOutTime
Definition: LA_GameFramework_classes.hpp:2794
class AFileLog * AILogFile
Definition: LA_GameFramework_classes.hpp:70
unsigned long bReachedPreciseRotation
Definition: LA_GameFramework_classes.hpp:2423
struct FBasedPosition LastMoveTargetPathLocation
Definition: LA_GameFramework_classes.hpp:2714
struct FVector LastOffsetAdjustment
Definition: LA_GameFramework_classes.hpp:2222
void SetFacePreciseRotation(const struct FRotator &RotationToFace, float InterpolationTime)
void ReachedIntermediateMoveGoal()
unsigned long bDrawDebugText
Definition: LA_GameFramework_classes.hpp:2569
struct FName InteractionTag
Definition: LA_GameFramework_classes.hpp:770
float MinDistance
Definition: LA_GameFramework_classes.hpp:2099
bool HandlePathObstruction(class AActor *BlockedBy)
Definition: LA_Engine_structs.hpp:4475
float LastPitchAdjustment
Definition: LA_GameFramework_classes.hpp:2189
struct FName MoveSyncGroupName
Definition: LA_GameFramework_classes.hpp:419
float BeaconMaxDist
Definition: LA_GameFramework_classes.hpp:314
void RecordDemoAILog(const struct FString &LogText)
TArray< struct FBehaviorEntry > TakeDamageBehaviors
Definition: LA_GameFramework_classes.hpp:331
TArray< struct FGameplayEventMetaData > AggregateEvents
Definition: LA_GameFramework_classes.hpp:2500
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1196
TArray< struct FName > DeathAnimNames
Definition: LA_GameFramework_classes.hpp:414
Definition: LA_Engine_classes.hpp:34866
unsigned long bKillWhenReached
Definition: LA_GameFramework_classes.hpp:741
Definition: LA_Engine_classes.hpp:4420
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:551
Definition: LA_GameFramework_classes.hpp:956
TArray< struct FBehaviorEntry > GroupWaitingBehaviors
Definition: LA_GameFramework_classes.hpp:335
void DrawDebug(class AHUD *H, const struct FName &Category)
unsigned long bPauseCrowd
Definition: LA_GameFramework_classes.hpp:858
class UClass * ThirdPersonCameraClass
Definition: LA_GameFramework_classes.hpp:2264
struct FVector HitLocationFromPhysMaterialTrace
Definition: LA_GameFramework_classes.hpp:1043
class UDynamicLightEnvironmentComponent * LightEnvironment
Definition: LA_GameFramework_classes.hpp:254
unsigned long bIsPlayingImportantAnimation
Definition: LA_GameFramework_classes.hpp:427
void SecondarySelect(const struct FVector &HitLoc, const struct FVector &HitNormal, const struct FTraceHitInfo &HitInfo)
TArray< class USeqEvent_HudRender * > KismetRenderEvents
Definition: LA_GameFramework_classes.hpp:1194
void ReachedPrecisePosition()
void NotifyCrowdAgentInRadius(class AGameCrowdAgent *Agent)
void DisplayDebug(class AHUD *HUD, float *out_YL, float *out_YPos)
void OnPlayAgentAnimation(class USeqAct_PlayAgentAnimation *Action)
float MaxSpeedBlendChangeSpeed
Definition: LA_GameFramework_classes.hpp:418
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:695
void SetFocusOnLoc(const struct FVector &FocusWorldLoc, const struct FVector2D &InterpSpeedRange, const struct FVector2D &InFocusFOV, float CameraFOV, bool bAlwaysFocus, bool bAdjustCamera, bool bIgnoreTrace, float FocusPitchOffsetDeg)
struct FGameEvents AllGameEvents
Definition: LA_GameFramework_classes.hpp:2502
void PrioritizeSpawnPoints(float DeltaTime, struct FCrowdSpawnInfoItem *Item)
float ScreenX
Definition: LA_GameFramework_classes.hpp:1680
void ActivatedBy(class AActor *NewActionTarget)
void PlayDeath(const struct FVector &KillMomentum)
Definition: LA_Core_structs.hpp:369
unsigned long bDoingDirectLook
Definition: LA_GameFramework_classes.hpp:2205
void InitNavigationHandle()
float CamShakeFalloff
Definition: LA_GameFramework_classes.hpp:1007
Definition: LA_GameFramework_structs.hpp:483
Definition: LA_AkAudio_classes.hpp:11
class AGameCrowdDestinationQueuePoint * QueuePosition
Definition: LA_GameFramework_classes.hpp:624
void ResetFacePreciseRotation()
float LightInitialBrightness
Definition: LA_GameFramework_classes.hpp:1035
class AGameCrowdDestinationQueuePoint * QueueHead
Definition: LA_GameFramework_classes.hpp:761
struct FVector2D CringeDuration
Definition: LA_GameFramework_classes.hpp:985
class UClass * CameraLensEffect
Definition: LA_GameFramework_classes.hpp:2791
void SetFocusOnActor(class AActor *FocusActor, const struct FName &FocusBoneName, const struct FVector2D &InterpSpeedRange, const struct FVector2D &InFocusFOV, float CameraFOV, bool bAlwaysFocus, bool bAdjustCamera, bool bIgnoreTrace, float FocusPitchOffsetDeg)
Definition: LA_GameFramework_classes.hpp:841
float CurrentHoverHeight
Definition: LA_GameFramework_classes.hpp:2705
Definition: LA_GameFramework_classes.hpp:535
class UClass * DebugCameraControllerClass
Definition: LA_GameFramework_classes.hpp:220
struct FVector HitLocation
Definition: LA_GameFramework_classes.hpp:990
class UClass * QueueBehaviorClass
Definition: LA_GameFramework_classes.hpp:817
class UCameraShake * CamShake_Left
Definition: LA_GameFramework_classes.hpp:2785
Definition: LA_Engine_classes.hpp:19855
void SpecialMoveFlagsUpdated()
void SetReachPreciseDestination(const struct FVector &DestinationToReach, bool bCancel)
class AActor * PanicFocus
Definition: LA_GameFramework_classes.hpp:575
int STATIC_GetObjClassVersion()
unsigned long bAllowsSpawning
Definition: LA_GameFramework_classes.hpp:748
float DesiredHoverHeight
Definition: LA_GameFramework_classes.hpp:2671
class UTexture2D * DisplayTexture
Definition: LA_GameFramework_classes.hpp:3341
float MaxWalkingSpeed
Definition: LA_GameFramework_classes.hpp:310
Definition: LA_GameFramework_classes.hpp:939
TArray< struct FRecentInteraction > RecentInteractions
Definition: LA_GameFramework_classes.hpp:313
struct FVector AdjustViewOffset(class APawn *P, const struct FVector &Offset)
unsigned long bPotentialEncounter
Definition: LA_GameFramework_classes.hpp:265
void SpawnExplosionParticleSystem(class UParticleSystem *Template)
Definition: LA_Engine_classes.hpp:5864
Definition: LA_GameFramework_structs.hpp:404
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1884
struct FPointer VfTable_IInterface_NavigationHandle
Definition: LA_GameFramework_classes.hpp:844
void OnDeactivate(class APlayerController *PC)
struct FVector HitNormal
Definition: LA_GameFramework_classes.hpp:1685
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2365
bool CanOverrideMoveWith(const struct FName &NewMove)
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2127
void ReattachMeshWithoutBeingSeen()
unsigned long bIsRoundStarted
Definition: LA_GameFramework_classes.hpp:2474
TArray< struct FAICmdHistoryItem > CommandHistory
Definition: LA_GameFramework_classes.hpp:72
unsigned long bDebugChangedCameraMode
Definition: LA_GameFramework_classes.hpp:2206
Definition: LA_GameFramework_classes.hpp:3336
float MaxTargetAcquireTime
Definition: LA_GameFramework_classes.hpp:421
TArray< struct FBehaviorEntry > ReachedBehaviors
Definition: LA_GameFramework_classes.hpp:772
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:3323
struct FString URLToLoad
Definition: LA_GameFramework_classes.hpp:3300
Definition: LA_GameFramework_classes.hpp:482
Definition: LA_GameFramework_classes.hpp:1149
Definition: LA_Engine_classes.hpp:34567
Definition: LA_Engine_classes.hpp:7358
void AgentDestroyed(class AGameCrowdAgent *Agent)
TArray< struct FBehaviorEntry > RandomBehaviors
Definition: LA_GameFramework_classes.hpp:330
bool ShouldConstrainAspectRatio()
class UParticleSystem * ParticleEmitterTemplate
Definition: LA_GameFramework_classes.hpp:987
float GetDesiredFOV(class APawn *ViewedPawn)
void UpdateFocusPoint(class APawn *P)
float LoopTime
Definition: LA_GameFramework_classes.hpp:547
unsigned long bAttachExplosionEmitterToAttachee
Definition: LA_GameFramework_classes.hpp:963
float SpawnRate
Definition: LA_GameFramework_classes.hpp:1713
unsigned long bUseRootMotionVelocity
Definition: LA_GameFramework_classes.hpp:422
bool AllowTransitionTo(class UClass *AttemptCommand)
Definition: LA_Core_classes.hpp:19
Definition: LA_Engine_classes.hpp:11102
float AwareRadius
Definition: LA_GameFramework_classes.hpp:279
struct FVector2D DOF_RadiusDistRange
Definition: LA_GameFramework_classes.hpp:2360
Definition: LA_Engine_classes.hpp:11921
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:3373
void FellOutOfWorld(class UClass *dmgType)
void PickNewDestinationFor(class AGameCrowdAgent *Agent, bool bIgnoreRestrictions)
bool NativeInputKey(int ControllerId, const struct FName &Key, TEnumAsByte< EInputEvent > Event, float AmountDepressed, bool bGamepad)
float WorstLocBlockedPct
Definition: LA_GameFramework_classes.hpp:2171
void Render(class UCanvas *TargetCanvas, class AHUD *TargetHud)
class UReachSpec * CurrentSpec
Definition: LA_GameFramework_classes.hpp:2676
void ModifyPostProcessSettings(struct FPostProcessSettings *PP)
unsigned char UnknownData00[0x3]
Definition: LA_GameFramework_classes.hpp:716
bool STATIC_StaticGetPlayerInfo(TArray< struct FCrowdSpawnerPlayerInfo > *out_PlayerInfo)
void PropagateViralBehaviorTo(class AGameCrowdAgent *OtherAgent)
class AActor * SelectedActor
Definition: LA_GameFramework_classes.hpp:1118
unsigned long bDrawDebugText
Definition: LA_GameFramework_classes.hpp:2903
void ProcessViewRotation(float DeltaTime, struct FRotator *out_ViewRotation, struct FRotator *out_DeltaRot)
unsigned long bApplyDeltaViewOffset
Definition: LA_GameFramework_classes.hpp:2321
unsigned long bShowMotionDebug
Definition: LA_GameFramework_classes.hpp:1176
float MaxRunningSpeed
Definition: LA_GameFramework_classes.hpp:311
void GetSeamlessTravelActorList(bool bToEntry, TArray< class AActor * > *ActorList)
float StickStrength
Definition: LA_GameFramework_classes.hpp:2032
unsigned long bHitObstacle
Definition: LA_GameFramework_classes.hpp:262
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:626
bool AtCapacity(unsigned char CheckCnt)
unsigned long bOldPlayRecoil
Definition: LA_GameFramework_classes.hpp:2122
float DesiredGroupRadius
Definition: LA_GameFramework_classes.hpp:336
Definition: LA_Engine_classes.hpp:4541
unsigned long bAllowSkeletonUpdateChangeBasedOnTickResult
Definition: LA_GameFramework_classes.hpp:423
struct FTextureUVs TrackballTouchIndicatorUVs
Definition: LA_GameFramework_classes.hpp:1188
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:504
Definition: LA_GameFramework_classes.hpp:2072
int LoopCount
Definition: LA_GameFramework_classes.hpp:26
struct FVector LastActualOriginOffset
Definition: LA_GameFramework_classes.hpp:2177
int STATIC_GetObjClassVersion()
Definition: LA_GameFramework_structs.hpp:660
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1011
int STATIC_GetObjClassVersion()
bool QueueReachedBy(class AGameCrowdAgent *Agent, const struct FVector &TestPosition)
class AActor * LastViewTarget
Definition: LA_GameFramework_classes.hpp:2272
void OnAnimEnd(class UAnimNodeSequence *SeqNode, float PlayedTime, float ExcessTime)
Definition: LA_GameFramework_classes.hpp:2696
void UpdateDestinations(class AGameCrowdDestination *NewDestination)
void UpdateCamera(class APawn *P, class AGamePlayerCamera *CameraActor, float DeltaTime, struct FTViewTarget *OutVT)
unsigned long bFleeDestination
Definition: LA_GameFramework_classes.hpp:745
bool STATIC_HoverBackToMesh(class AGameAIController *AI)
void DrawMobileZone_Trackball(class UMobileInputZone *Zone)
void Render(class UCanvas *TargetCanvas, class AHUD *TargetHud)
struct FVector LocationOffset
Definition: LA_GameFramework_classes.hpp:25
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:602
unsigned long bIsPlayingIdleAnimation
Definition: LA_GameFramework_classes.hpp:425
unsigned long bBoneSpaceRecoil
Definition: LA_GameFramework_classes.hpp:2120
TArray< struct FName > WalkAnimNames
Definition: LA_GameFramework_classes.hpp:411
class UPlayer * OriginalPlayer
Definition: LA_GameFramework_classes.hpp:1116
float RadiusOfBehaviorEvent
Definition: LA_GameFramework_classes.hpp:713
int ObstacleCheckCount
Definition: LA_GameFramework_classes.hpp:304
unsigned long bBlendBetweenAnims
Definition: LA_GameFramework_classes.hpp:1859
Definition: LA_Engine_classes.hpp:9459
float ScreenY
Definition: LA_GameFramework_classes.hpp:1681
float PenetrationBlendInTime
Definition: LA_GameFramework_classes.hpp:2174
Definition: LA_Engine_classes.hpp:5693
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2082
float MinBehindSpawnDist
Definition: LA_GameFramework_classes.hpp:1716
float BlendOutTime
Definition: LA_GameFramework_classes.hpp:540
struct FVector DisplayLocation
Definition: LA_GameFramework_classes.hpp:3369
float BlendOutTime
Definition: LA_GameFramework_classes.hpp:1855
unsigned long bHasExploded
Definition: LA_GameFramework_classes.hpp:1027
bool STATIC_MustBeHiddenFromThisPoint(class UNavigationHandle *NavHandle, const struct FVector &InOutOfViewLocation)
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:860
float InitialLastRenderTime
Definition: LA_GameFramework_classes.hpp:341
void InitBehavior(class AGameCrowdAgent *Agent)
int MaxRoundNumber
Definition: LA_GameFramework_classes.hpp:2476
Definition: LA_GameFramework_classes.hpp:3318
unsigned long bLockedToViewTarget
Definition: LA_GameFramework_classes.hpp:2310
struct FTextureUVs SliderUVs[0x4]
Definition: LA_GameFramework_classes.hpp:1190
struct FVector DisplayLocation
Definition: LA_GameFramework_classes.hpp:3340
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:28
void DrawInputZoneOverlays()
unsigned long bAllowAsPreviousDestination
Definition: LA_GameFramework_classes.hpp:742
float FollowingInterpSpeed_Pitch
Definition: LA_GameFramework_classes.hpp:2326
TArray< struct FBehaviorEntry > SeePlayerBehaviors
Definition: LA_GameFramework_classes.hpp:323
class UPointLightComponent * ExplosionLight
Definition: LA_GameFramework_classes.hpp:1031
class USkeletalMeshComponent * SkeletalMeshComponent
Definition: LA_GameFramework_classes.hpp:404
TArray< struct FBehaviorEntry > UneasyBehaviors
Definition: LA_GameFramework_classes.hpp:327
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1664
void ReachedDestination(class AGameCrowdAgent *Agent)
TArray< class UClass * > RestrictedAgentClasses
Definition: LA_GameFramework_classes.hpp:767
Definition: LA_Core_classes.hpp:624
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2571
class AGameCrowdAgent * QueryingAgent
Definition: LA_GameFramework_classes.hpp:854
void NotifySeePlayer(class APlayerController *PC)
void OnBecomeInActive(class UGameCameraBase *NewCamera)
Definition: LA_GameFramework_classes.hpp:1677
class AController * InstigatorController
Definition: LA_GameFramework_classes.hpp:1041
float TurnCurTime
Definition: LA_GameFramework_classes.hpp:2208
float DeltaRoll
Definition: LA_GameFramework_classes.hpp:1946
float WorstLocInterpSpeed
Definition: LA_GameFramework_classes.hpp:2216
unsigned long bRequireLOS
Definition: LA_GameFramework_classes.hpp:717
Definition: LA_Engine_classes.hpp:2183
TArray< struct FRequiredMobileInputConfig > RequiredMobileInputConfigs
Definition: LA_GameFramework_classes.hpp:44
Definition: LA_GameFramework_classes.hpp:178
Definition: LA_GameFramework_classes.hpp:2663
class UGameAICommand * GetActiveCommand()
struct FColor DisplayColor
Definition: LA_GameFramework_classes.hpp:3368
unsigned long bDoingACameraTurn
Definition: LA_GameFramework_classes.hpp:2202
float ViralRadius
Definition: LA_GameFramework_classes.hpp:496
unsigned long bLastHitWasHeadShot
Definition: LA_GameFramework_classes.hpp:1085
TArray< struct FName > RunAnimNames
Definition: LA_GameFramework_classes.hpp:412
unsigned long bFocusPointSuccessful
Definition: LA_GameFramework_classes.hpp:2201
class AActor * MoveToActor
Definition: LA_GameFramework_classes.hpp:2713
void PrePushed(class AGameAIController *AI)
void STATIC_ShowLoadingMovie(bool bShowMovie, bool bPauseAfterHide, float PauseDuration, float KeepPlayingDuration, bool bOverridePreviousDelays)
void ForcePawnRotation(class APawn *P, const struct FRotator &NewRotation)
class UNavigationHandle * NavigationHandle
Definition: LA_GameFramework_classes.hpp:303
float FollowingCameraVelThreshold
Definition: LA_GameFramework_classes.hpp:2329
Definition: LA_GameFramework_classes.hpp:2856
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:926
float LastHeightAdjustment
Definition: LA_GameFramework_classes.hpp:2188
float CamShakeOuterRadius
Definition: LA_GameFramework_classes.hpp:2789
struct FVector2D InitialTouch
Definition: LA_GameFramework_classes.hpp:2100
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:223
void InitSpecialMoveFlags(int *out_Flags)
struct FVector LastPreModifierCameraLoc
Definition: LA_GameFramework_classes.hpp:2219
Definition: LA_GameFramework_classes.hpp:2814
Definition: LA_Core_structs.hpp:245
unsigned long bAILogToWindow
Definition: LA_GameFramework_classes.hpp:65
void InitializeAgent(class AActor *SpawnLoc, class AGameCrowdAgent *AgentTemplate, class UGameCrowdGroup *NewGroup, float AgentWarmupTime, bool bWarmupPosition, bool bCheckWarmupVisibility, TArray< struct FCrowdSpawnerPlayerInfo > *PlayerInfo)
struct FDamageEvents AllDamageEvents
Definition: LA_GameFramework_classes.hpp:2508
unsigned long bCausesFracture
Definition: LA_GameFramework_classes.hpp:965
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:433
class UCameraShake * CamShake_Right
Definition: LA_GameFramework_classes.hpp:1003
bool STATIC_HoverToPoint(class AGameAIController *AI, const struct FVector &InPoint, float InGoalDistance, float InHoverHeight)
struct FVector WorldToRelativeOffset(const struct FRotator &InRotation, const struct FVector &WorldSpaceOffset)
Definition: LA_GameFramework_structs.hpp:497
Definition: LA_GameFramework_classes.hpp:922
void ActivateBehavior(class UGameCrowdAgentBehavior *NewBehaviorArchetype, class AActor *LookAtActor)
struct FName CurrentSoundMode
Definition: LA_GameFramework_classes.hpp:185
unsigned long bWantsSeePlayerNotification
Definition: LA_GameFramework_classes.hpp:260
unsigned long bFollowTarget
Definition: LA_GameFramework_classes.hpp:2312
unsigned long bFocusPointSet
Definition: LA_GameFramework_classes.hpp:2200
int CurrentConformTraceInterval
Definition: LA_GameFramework_classes.hpp:277
struct FWeaponEvents AllWeaponEvents
Definition: LA_GameFramework_classes.hpp:2505
unsigned long bClearOldArchetypes
Definition: LA_GameFramework_classes.hpp:1705
float DirectionalExplosionMinDot
Definition: LA_GameFramework_classes.hpp:1046
TArray< class UObject * > Targets
Definition: LA_GameFramework_classes.hpp:1900
class AActor * ActorToIgnoreForDamage
Definition: LA_GameFramework_classes.hpp:978
struct FVector IntermediatePoint
Definition: LA_GameFramework_classes.hpp:2708
class AController * AttacheeController
Definition: LA_GameFramework_classes.hpp:1045
Definition: LA_Engine_classes.hpp:11281
Definition: LA_GameFramework_classes.hpp:3364
int MaxAgents
Definition: LA_GameFramework_classes.hpp:1712
float SeePlayerInterval
Definition: LA_GameFramework_classes.hpp:325
float Damage
Definition: LA_GameFramework_classes.hpp:975
unsigned long bIgnoreInstigator
Definition: LA_GameFramework_classes.hpp:960
unsigned long bTemporaryOriginRotInterp
Definition: LA_GameFramework_classes.hpp:2397
float AvoidOtherRadius
Definition: LA_GameFramework_classes.hpp:280
class UAnimNodeSequence * ActionSeqNode
Definition: LA_GameFramework_classes.hpp:407
void FinishedTargetRotation()
float MomentumTransferScale
Definition: LA_GameFramework_classes.hpp:986
bool STATIC_MoveToGoal(class AGameAIController *AI, class AActor *InGoal, float InGoalDistance, float InHoverHeight)
void Tick(float DeltaTime)
unsigned long bSpawnAtEdge
Definition: LA_GameFramework_classes.hpp:752
float SpeedBlendEnd
Definition: LA_GameFramework_classes.hpp:416
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:673
Definition: LA_Engine_structs.hpp:4210
unsigned long bAllowTeammateCringes
Definition: LA_GameFramework_classes.hpp:961
Definition: LA_Engine_classes.hpp:7688
Definition: LA_Engine_classes.hpp:11736
float U
Definition: LA_GameFramework_classes.hpp:3344
TArray< struct FPenetrationAvoidanceFeeler > PenetrationAvoidanceFeelers
Definition: LA_GameFramework_classes.hpp:2221
struct FCamFocusPointParams FocusPoint
Definition: LA_GameFramework_classes.hpp:2199
Definition: LA_GameFramework_classes.hpp:2743
TArray< class UObject * > RestrictedArchetypes
Definition: LA_GameFramework_classes.hpp:768
struct FColor ButtonCaptionColor
Definition: LA_GameFramework_classes.hpp:1184
float TimeToBecomeViral
Definition: LA_GameFramework_classes.hpp:498
unsigned long bUseMapSpecificValues
Definition: LA_GameFramework_classes.hpp:969
float CameraLensEffectRadius
Definition: LA_GameFramework_classes.hpp:2792
Definition: LA_Basic.hpp:22
float RandomBehaviorInterval
Definition: LA_GameFramework_classes.hpp:332
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:122
unsigned long bSmoothViewOffsetPitchChanges
Definition: LA_GameFramework_classes.hpp:2320
unsigned long bAllowVisibleSpawning
Definition: LA_GameFramework_classes.hpp:750
float CamShakeOuterRadius
Definition: LA_GameFramework_classes.hpp:1006
TArray< struct FBehaviorEntry > SpawnBehaviors
Definition: LA_GameFramework_classes.hpp:326
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:3302
void ActivateInstancedBehavior(class UGameCrowdAgentBehavior *NewBehaviorObject)
TArray< struct FCrowdSpawnInfoItem > ScriptedSpawnInfo
Definition: LA_GameFramework_classes.hpp:846
struct FName PrimaryKey
Definition: LA_GameFramework_classes.hpp:1108
void Paused(class UGameAICommand *NewCommand)
struct FVector GeneratePathToActor(class AActor *Goal, float WithinDistance, bool bAllowPartialPath)
float EyeZOffset
Definition: LA_GameFramework_classes.hpp:295
bool STATIC_InitCommand(class AGameAIController *AI)
struct FRotator LastIdealCameraOriginRot
Definition: LA_GameFramework_classes.hpp:2184
int Capacity
Definition: LA_GameFramework_classes.hpp:762
bool MessageEvent(const struct FName &EventName, class UObject *Sender)
float ReachThreshold
Definition: LA_GameFramework_classes.hpp:334
Definition: LA_GameFramework_classes.hpp:620
TArray< struct FPlayerEvents > AllPlayerEvents
Definition: LA_GameFramework_classes.hpp:2504
class UCameraShake * CamShake_Left
Definition: LA_GameFramework_classes.hpp:1002
Definition: LA_GameFramework_classes.hpp:1638
void ClearQueue(class AGameCrowdAgent *OldCustomer)
void PostRender(class UCanvas *Canvas)
Definition: LA_GameFramework_classes.hpp:1965
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1904
static UClass * FindClass(const std::string &name)
Definition: LA_Core_classes.hpp:72
float MobileTiltX
Definition: LA_GameFramework_classes.hpp:1191
unsigned long bShowDebug
Definition: LA_GameFramework_classes.hpp:1576
void Touch(class AActor *Other, class UPrimitiveComponent *OtherComp, const struct FVector &HitLocation, const struct FVector &HitNormal)
unsigned char UnknownData00[0x3]
Definition: LA_GameFramework_classes.hpp:2395
void GetSpawnPosition(class USeqAct_GameCrowdSpawner *Spawner, struct FVector *SpawnPos, struct FRotator *SpawnRot)
Definition: LA_Engine_classes.hpp:10731
unsigned long bLooping
Definition: LA_GameFramework_classes.hpp:1858
class AGameCrowdDestination * QueueDestination
Definition: LA_GameFramework_classes.hpp:813
class UGameThirdPersonCameraMode * CurrentCamMode
Definition: LA_GameFramework_classes.hpp:2187
class UAnimNodeSlot * FullBodySlot
Definition: LA_GameFramework_classes.hpp:406
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2104
float GetDestinationRadius()
void FinishedTargetRotation()
unsigned long bInterpRotation
Definition: LA_GameFramework_classes.hpp:2315
TArray< struct FName > AnimationList
Definition: LA_GameFramework_classes.hpp:1853
unsigned long bUsePerAxisOriginLocInterp
Definition: LA_GameFramework_classes.hpp:2314
void ExtractSpecialMoveFlags(int Flags)
struct FVector OutOfViewLocation
Definition: LA_GameFramework_classes.hpp:1575
void SetFreezeRendering()
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1687
Definition: LA_Engine_classes.hpp:32877
unsigned long bRespondToExplosions
Definition: LA_GameFramework_classes.hpp:1086
float StrafeOffsetInterpSpeedIn
Definition: LA_GameFramework_classes.hpp:2336
class UGameCameraBase * CurrentCamera
Definition: LA_GameFramework_classes.hpp:2267
float BlendInTime
Definition: LA_GameFramework_classes.hpp:539
struct FVector ExplosionDirection
Definition: LA_GameFramework_classes.hpp:1047
int NumMovePointFails
Definition: LA_GameFramework_classes.hpp:2710
struct FString GetActionString()
void WarmupPause(bool bDesiredPauseState)
void ProcessViewRotation(float DeltaTime, class AActor *ViewTarget, struct FRotator *out_ViewRotation, struct FRotator *out_DeltaRot)
Definition: LA_GameFramework_classes.hpp:2566
void HandlePotentialAgentEncounter()
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:907
int DirectLookYaw
Definition: LA_GameFramework_classes.hpp:2214
void ResetInterpolation()
class UCameraShake * CamShake_Rear
Definition: LA_GameFramework_classes.hpp:1004
float LastDOFRadius
Definition: LA_GameFramework_classes.hpp:2354
Definition: LA_GameFramework_classes.hpp:2494
class AGameCrowdAgent * AgentEnRoute
Definition: LA_GameFramework_classes.hpp:773
struct FVector IntermediatePoint
Definition: LA_GameFramework_classes.hpp:300
unsigned long bIsBeyondSpawnDistance
Definition: LA_GameFramework_classes.hpp:757
void DisableDebugCamera()
class UStaticMeshComponent * Mesh
Definition: LA_GameFramework_classes.hpp:460
Definition: LA_Core_structs.hpp:710
void ClientColorFade(const struct FColor &FadeColor, unsigned char FromAlpha, unsigned char ToAlpha, float FadeTime)
float V
Definition: LA_GameFramework_classes.hpp:3345
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1722
Definition: LA_GameFramework_classes.hpp:108
Definition: LA_GameFramework_classes.hpp:1940
void AddSpawnPoint(class AGameCrowdDestination *GCD)
struct FProjectileEvents AllProjectileEvents
Definition: LA_GameFramework_classes.hpp:2506
unsigned long bReachedPreciseDestination
Definition: LA_GameFramework_classes.hpp:2421
class UClass * ActorClassToIgnoreForDamage
Definition: LA_GameFramework_classes.hpp:979
TEnumAsByte< ECrowdBehaviorEvent > ViralBehaviorEvent
Definition: LA_GameFramework_classes.hpp:486
Definition: LA_Engine_classes.hpp:6617
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2801
unsigned long bFaceActionTargetFirst
Definition: LA_GameFramework_classes.hpp:491
unsigned long bLastCanDoSpecialMove
Definition: LA_GameFramework_classes.hpp:2419
class UFont * ButtonFont
Definition: LA_GameFramework_classes.hpp:1183
struct FString GetDebugVerboseText()
TArray< class AActor * > TouchedActors
Definition: LA_GameFramework_classes.hpp:2101
TEnumAsByte< ECameraViewportTypes > CurrentViewportType
Definition: LA_GameFramework_classes.hpp:2363
float MaxPlayerDistance
Definition: LA_GameFramework_classes.hpp:495
bool InternalCanDoSpecialMove()
class AGameCrowdDestination * BehaviorDestination
Definition: LA_GameFramework_classes.hpp:249
struct FViewOffsetData ViewOffset
Definition: LA_GameFramework_classes.hpp:2347
unsigned long bSoftPerimeter
Definition: LA_GameFramework_classes.hpp:753
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2478
TArray< struct FVector > LocationsToCheck
Definition: LA_GameFramework_classes.hpp:1601
float MaxPathLaneValue
Definition: LA_GameFramework_classes.hpp:288
bool AbortCommand(class UGameAICommand *AbortCmd, class UClass *AbortClass)
float TemporaryOriginRotInterpSpeed
Definition: LA_GameFramework_classes.hpp:2398
struct FPointer VfTable_IEditorLinkSelectionInterface
Definition: LA_GameFramework_classes.hpp:740
Definition: LA_GameFramework_classes.hpp:2028
void AdjustTurn(int AngleOffset)
float DeltaPitch
Definition: LA_GameFramework_classes.hpp:1947
float ExploRadialBlurFadeOutTime
Definition: LA_GameFramework_classes.hpp:997
void AdvanceCustomerTo(class AGameCrowdInteractionPoint *FrontPosition)
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:1642
class UAnimNodeSequence * WalkSeqNode
Definition: LA_GameFramework_classes.hpp:408
unsigned long bPendingPop
Definition: LA_GameFramework_classes.hpp:120
class UParticleSystem * ParticleEmitterTemplate
Definition: LA_GameFramework_classes.hpp:2798
int CommandHistoryNum
Definition: LA_GameFramework_classes.hpp:73
float VisibleProximityLODDist
Definition: LA_GameFramework_classes.hpp:297
struct FName Handle
Definition: LA_GameFramework_classes.hpp:2417
struct FString GetBehaviorString()
unsigned long bFillPotentialSpawnPoints
Definition: LA_GameFramework_classes.hpp:1708
class AGameCrowdAgent * QueuedAgent
Definition: LA_GameFramework_classes.hpp:812
float GoalDistance
Definition: LA_GameFramework_classes.hpp:2674
Definition: LA_GameFramework_classes.hpp:2466
class UGameStateObject * GameState
Definition: LA_GameFramework_classes.hpp:2497
void ActivatedBy(class AActor *NewActionTarget)
Definition: LA_Engine_classes.hpp:11156
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2431
void UnTouch(class AActor *Other)
int AnimationIndex
Definition: LA_GameFramework_classes.hpp:549
void OnToggle(class USeqAct_Toggle *Action)
void OnToggleMouseCursor(class USeqAct_ToggleMouseCursor *inAction)
void OnDeactivate(class APlayerController *PC)
Definition: LA_GameFramework_classes.hpp:1595
class UAudioComponent * AmbientSoundComponent
Definition: LA_GameFramework_classes.hpp:319
void ShowDebugSelectedInfo()
float DurationOfBehavior
Definition: LA_GameFramework_classes.hpp:488
void OnActivate(class APlayerController *PC)
void PlayerUpdateCamera(class APawn *P, class AGamePlayerCamera *CameraActor, float DeltaTime, struct FTViewTarget *OutVT)
unsigned long bAILogging
Definition: LA_GameFramework_classes.hpp:64
float LastPathingAttempt
Definition: LA_GameFramework_classes.hpp:306
float PenetrationBlendOutTime
Definition: LA_GameFramework_classes.hpp:2173
bool SetFocusPoint(class APawn *ViewedPawn)
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:2839
void SpawnCameraLensEffects()
TArray< struct FAgentArchetypeInfo > ListOfAgents
Definition: LA_GameFramework_classes.hpp:2746
struct FCrowdSpawnInfoItem CloudSpawnInfo
Definition: LA_GameFramework_classes.hpp:845
struct FVector LastRunOffset
Definition: LA_GameFramework_classes.hpp:2344
void UpdateAllSpawners(float DeltaTime)
class UPointLightComponent * ExploLight
Definition: LA_GameFramework_classes.hpp:2793
Definition: LA_Engine_classes.hpp:1945
Definition: LA_Engine_structs.hpp:3699
void DrawMobileZone_Joystick(class UMobileInputZone *Zone)
void DrawDebug(class AHUD *H, const struct FName &Category)
bool DisplayMaterials(float X, float DY, class UMeshComponent *MeshComp, float *Y)
float DOF_FocusInnerRadius
Definition: LA_GameFramework_classes.hpp:2351
int ExtraPathCost
Definition: LA_GameFramework_classes.hpp:290
void AddToMobileInput(class UMobilePlayerInput *MPI)
int StartOfRenderingMovieFrame
Definition: LA_GameFramework_classes.hpp:1661
Definition: LA_GameFramework_classes.hpp:241
void PatchDebugCameraController()
unsigned long bUseNavMeshPathing
Definition: LA_GameFramework_classes.hpp:259
struct FName Status
Definition: LA_GameFramework_classes.hpp:114
void TeleportPawnToCamera(bool bToggleDebugCameraOff)
struct FName ChildStatus
Definition: LA_GameFramework_classes.hpp:112
Definition: LA_Engine_classes.hpp:1254
float PENALTY_COEFF_ANGLETOGOAL
Definition: LA_GameFramework_classes.hpp:282
void AddMember(class AGameCrowdAgent *Agent)
unsigned long bDrawDebugText
Definition: LA_GameFramework_classes.hpp:1112
class AGameCrowdBehaviorPoint * STATIC_TriggerCrowdBehavior(TEnumAsByte< ECrowdBehaviorEvent > EventType, class AActor *Instigator, const struct FVector &AtLocation, float InRange, float InDuration, class AActor *BaseActor, bool bRequireLOS)
Definition: LA_GameFramework_structs.hpp:504
float DebugTextMaxLen
Definition: LA_GameFramework_classes.hpp:71
unsigned long bTrackExplosionParticleSystemLifespan
Definition: LA_GameFramework_classes.hpp:1029
float MaxLOSLifeDistanceSq
Definition: LA_GameFramework_classes.hpp:338
struct FString GetBehaviorString()
static UClass * StaticClass()
Definition: LA_GameFramework_classes.hpp:463
float CamShakeInnerRadius
Definition: LA_GameFramework_classes.hpp:2788
struct FVector GetCollisionExtent()
unsigned long bIsInSpawnPool
Definition: LA_GameFramework_classes.hpp:271
unsigned long bAllowNewSameClassInstance
Definition: LA_GameFramework_classes.hpp:115
Definition: LA_GameFramework_classes.hpp:2413
void DrawMobileZone_Slider(class UMobileInputZone *Zone)
bool HandlePathObstruction(class AActor *BlockedBy)
class UTexture2D * JoystickHat
Definition: LA_GameFramework_classes.hpp:1179
unsigned long bOrientCameraShakeTowardsEpicenter
Definition: LA_GameFramework_classes.hpp:2782
float KnockDownRadius
Definition: LA_GameFramework_classes.hpp:982
unsigned long bIsEnabled
Definition: LA_GameFramework_classes.hpp:692
float ForcedCamFOV
Definition: LA_GameFramework_classes.hpp:2271
struct FPointer GoalPoly
Definition: LA_GameFramework_classes.hpp:1574
unsigned long bBadHitNormal
Definition: LA_GameFramework_classes.hpp:263
struct FVector PendingVelocity
Definition: LA_GameFramework_classes.hpp:247
TArray< struct FAggregateEventMapping > AggregatesList
Definition: LA_GameFramework_classes.hpp:2498
int LoopIndex
Definition: LA_GameFramework_classes.hpp:546
float GetEffectCheckRadius(bool bCauseDamage, bool bCauseFractureEffects, bool bCauseEffects)
Definition: LA_Core_structs.hpp:513
class UTexture2D * TrackballTouchIndicator
Definition: LA_GameFramework_classes.hpp:1187
unsigned long bRotInterpSpeedConstant
Definition: LA_GameFramework_classes.hpp:2316
bool IsEnemyBasedOnInterpActor(class APawn *InEnemy)
float FollowingInterpSpeed_Roll
Definition: LA_GameFramework_classes.hpp:2328
unsigned long bWasFiring
Definition: LA_GameFramework_classes.hpp:2670
bool CanOverrideSpecialMove(const struct FName &InMove)
struct FString GetBehaviorString()
class UClass * PhysicalMaterialPropertyClass
Definition: LA_GameFramework_classes.hpp:3321
void SetCrowdInfoVolume(class AGameCrowdInfoVolume *Vol)
unsigned long bUseRootMotion
Definition: LA_GameFramework_classes.hpp:541
Definition: LA_Core_structs.hpp:560
struct FMap_Mirror AggregateEventsMapping
Definition: LA_GameFramework_classes.hpp:2499